Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial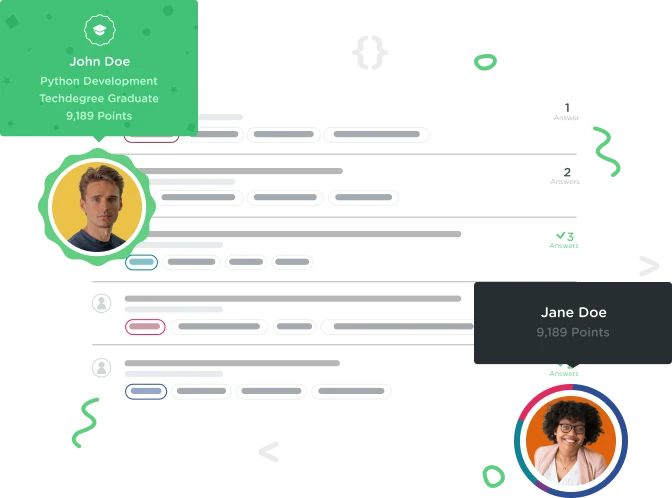
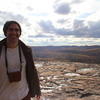
mannyaharon
873 PointsDid you add a constructor that takes a String?
I am almost certain I did exactly what the task asks. Any ideas as to why I am getting the error "Did you add a constructor that takes a String?"
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public String theTopic(String topic){
return mTopic = topic;
}
public void addPost(ForumPost post) {
/* When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
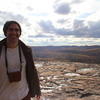
mannyaharon
873 PointsI just did. Sorry about that! Thanks for the quick response.
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public String theTopic(String topic){
return mTopic = topic;
}
public void addPost(ForumPost post) {
/* When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
2 Answers

Chase Marchione
155,055 PointsHi Emanuel,
You'll want the constructor to have the same name as the class (instead of 'theTopic'.)
Since you're setting the value of the variable, rather than returning a value, you don't need the return keyword.
public Forum(String topic) {
mTopic = topic;
}
Hope this helps!
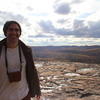
mannyaharon
873 PointsThanks! Although that works, I am a bit unsettled because I don't understand why your code works as opposed to mine. I've really been struggling to fully grasp all this. Would you happen to know of other resources that may help me fully understand the functionality/purpose/scope of class, methods, constructors and the like?
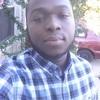
josue exhume
20,981 PointsCJ Marchione is right, i just want to point out, one common practice trend is to setup a getter and setter method.
public class Forum {
private String mTopic;
public String getTopic() { // this is our getter , ie "get"topic
return mTopic;
}
public void setTopic(String mTopic){ // this is our setter, ie "set"topic
this.mTopic = mTopic;
}
public Forum (String topic) { // this is our constructor
setTopic(topic);
}
public void addPost(ForumPost post) {
/* When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
Seth Kroger
56,413 PointsSeth Kroger
56,413 PointsCan you post your code?