Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial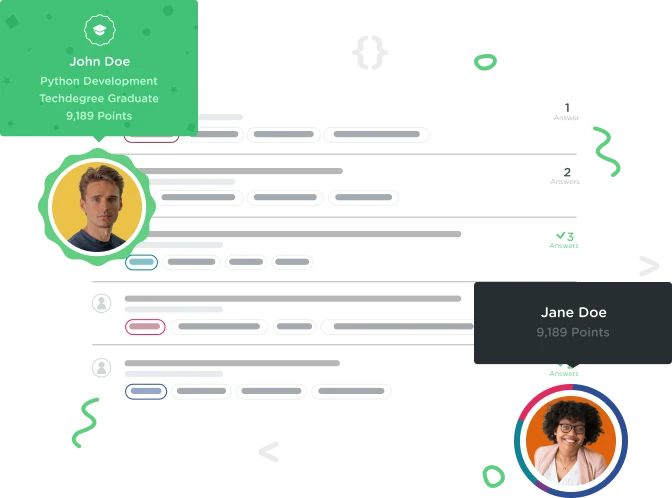
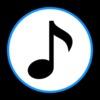
Daniel Irchai
7,260 PointsDid you declare the 'lapTimes' variable as a 'double[]' or 'var'? Um...What?
The first task of this Code Challenge asked me to declare an array of doubles named lapTimes with a length of 4. But then I got a 'Bummer!', asking whether I declared the array with 'double' or 'var'. But I declared it with 'double', which is what I thought they wanted. So I then tried replacing 'double' with 'var', but the same 'Bummer!' message popped up. What am I doing wrong here?
double []lapTimes = new[4]
2 Answers

andren
28,558 PointsThere are three issues:
The task is a bit picky about how your code is formatted. It wants the square brackets [] to be placed right after the word
double
without any spaces between them. Either way is technically valid in C# but you have to go with that the challenge is expecting if you want your code to pass.You have to include the word
double
after the wordnew
in your code. This is not just a quirk of the challenge checker but an actual C# requirement. Your code would not compile without specifying what type of object you are creating with thenew
keyword.You are not terminating the line with a semicolon.
If you fix those three issues like this
double[] lapTimes = new double[4];
Then your code will work.

Keenan Johnson
13,841 PointsWhen declaring an array, the syntax you want is datatype[]. Also, when defining the array length, the syntax you want is datatype[length].
Example:
double[] lapTimes = new double[20];
Daniel Irchai
7,260 PointsDaniel Irchai
7,260 PointsIt worked! Thanks for the help. But it was mentioned in the video that preceded this challenge that double wasn't always needed to be put after the word new. They said that the computer would be able to identify what type of object was in the array just by seeing what the array contained. Was the task just pick, or did I misunderstand something? Regardless, the code worked, so thanks again.
andren
28,558 Pointsandren
28,558 PointsThat is true, but in order to identify the type based on what is in the array, there actually needs to be something in the array.
In other words when you declare an array and specify some items at the same time like this:
double[] lapTimes = new[] {2.4, 3.1, 1.4};
Then it is fine to not specify the type and size of the array. But when the array is empty, which the array you make in this task is. Then you have to tell C# what type the array contains, since there is no content to base it on. That is what I meant to convey in my original post.