Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial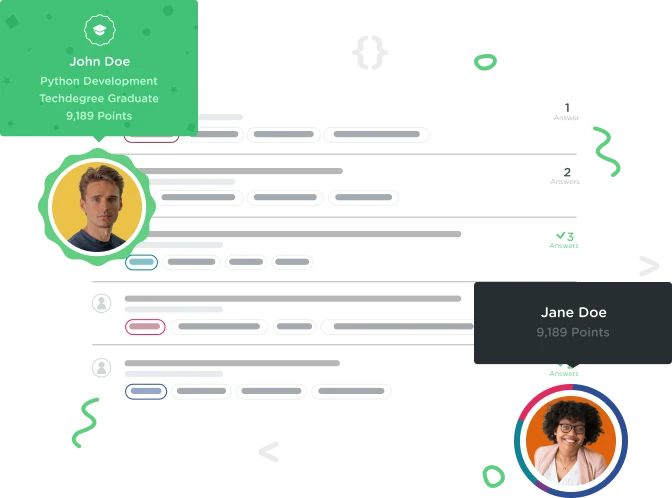

Kyle Salisbury
Full Stack JavaScript Techdegree Student 16,363 PointsDid you forget to create the method getTileCount that accepts a char? I feel like I have but it keeps saying I haven't.
public String getTileCount() { int tileCount =0; for(char tile: mHand.toCharArray()) { if (mHand.indexOf(tile)>=0) { tileCount++; } } String cont = Integer.toString(tileCount); return count; }
Maybe i'm just missing the concept or the question but I can't get past this one.
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public String getTileCount() {
int tileCount = 0;
for (char tile: mHand.toCharArray()) {
if (mHand.indexOf(tile) >=0) {
tileCount++;
}
}
String count = Integer.toString(tileCount);
return count;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
}
2 Answers
Christopher Augg
21,223 PointsHello Kyle, I am not sure if you got this answered yet so I will try and help you out.
The question is asking you to use a for each loop to loop through the mHand Stirng and test each character in that string against a character passed into the method. With characters we can just use the == operator unlike with Strings where we need to use string.equals(string). One way to do this is with the following:
public int getTileCount(char ch) {
int count = 0;
for (char c : mHand.toCharArray()) {
if(c == ch) {
count++;
}
}
return count;
}
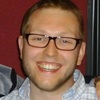
Victor Learned
6,976 PointsYour public String getTileCount() is currently not taking ANY variables let alone a char. Thus you aren't actually tell your method what char it should be searching for. Trying something like:
public String getTileCount(char c)
{
int tileCount = 0;
for (char tile: mHand.toCharArray()) {
if (tile == c)) {
tileCount++;
}
}
return tileCount;
}

Kyle Salisbury
Full Stack JavaScript Techdegree Student 16,363 PointsThanks for responding so quick! However with that suggestion i get two errors:
./ScrabblePlayer.java:21: error: char cannot be dereferenced if (tile.equals(c)) { ^ ./ScrabblePlayer.java:26: error: incompatible types: int cannot be converted to String return tileCount; ^ 2 errors
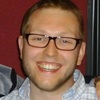
Victor Learned
6,976 PointsYep some reason was thinking a string. Though you could turn it into a Character object and do a .equals compare :)
Kyle Salisbury
Full Stack JavaScript Techdegree Student 16,363 PointsKyle Salisbury
Full Stack JavaScript Techdegree Student 16,363 PointsThanks man, that was it. I don't really understand the c == ch part but i'll keep studying a bit and maybe it will catch on for me. appreciate it!
Christopher Augg
21,223 PointsChristopher Augg
21,223 PointsNo Problem..... The reason for the c == ch is because every character is also a number on the ASCII table. Here is a link to one : http://web.cs.mun.ca/~michael/c/ascii-table.html
In this current program we are specifically making two variables of type char and naming them c and ch. We could name them charOne and charTwo.
These two variables are of type char and hold characters (i.e a b c d e........). These characters are also numbers like I had mentioned (i.e 97 98 99 100 101......). Therefore, if charOne is assigned the character h and charTwo is assigned the character z, then when testing if(charOne == charTwo) will be false because we are essentially testing if 104 is equal to 122.
I hope that helps...