Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial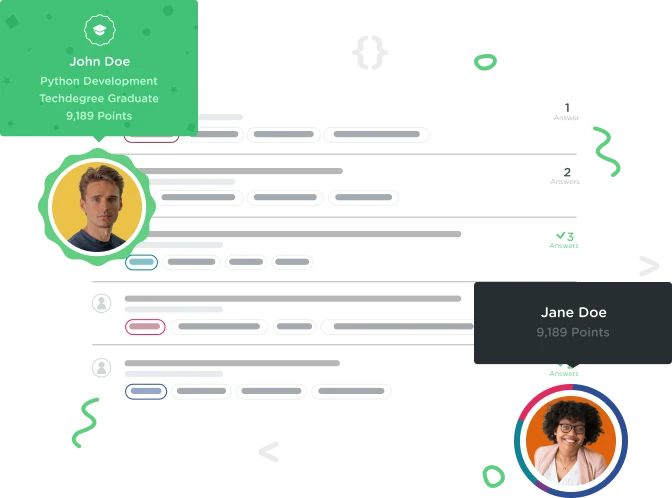

siddharth seshadri
158 Points"Did you forget to pass the `firstName` parameter to the printf function?" Getting this error in java beginner track
console.printf("First Name %s", console.readline(firstName)) what is wrong with this
// I have imported java.io.Console for you. It is a variable called console.
String firstName = "Siddharth";
String lastName = "Siddharth";
console.readLine(firstName);
console.readLine(lastName);
console.printf("First Name, %s",console.readLine(firstName));
4 Answers

Tabatha Trahan
21,422 PointsYou can consolidate your code. Try declaring your variable and then having it set to the input received through the console.readLine() method for both firstName and lastName:
String firstName = console.readLine();
String lastName = console.readLine();
console.printf("First name: %s", firstName);
To walk through this: first you are declaring a variable firstName of type String and setting it equal to the value received by console.readLine(). Then you are repeating for the lastName variable. Call the printf method using %s as a place holder and pass it the firstName variable which will be evaluated to the value that was obtained from the console.readLine method. Does that make sense? Then repeat for the last name part of the challenge. Let us know if you are still having problems with this one.

Tabatha Trahan
21,422 PointsIt looks like the issue is with how the printf method was called. The %s string placeholder is expecting a single string value to be passed to it. This can be done by passing it the firstName variable:
console.printf("First Name, %s", firstName);
The console.readLine() was used above your printf statement to get and store the value of firstName. I hope this helps.
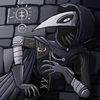
Toma Inashvili
634 PointsAssign firstName and lastName Strings to the console.readLine().
String firstName = console.readLine(); String lastName = console.readLine();
You don't need to enter your own name. The program is getting both first name and last name values from the user via console.readLine method. [However I do not know if task required to do it in the way you have done]
Then, try changing last line to console.printf("First name, %s", firstName)
Let me know if this helps

siddharth seshadri
158 PointsThank you for your reply toma I tried what you said and it still didn't work. Any other suggestions?
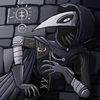
Toma Inashvili
634 PointsThe 'error' I have noticed is that you used comma after First name in the console.printf method. However I have seen the task and it requires to write : after First name. [I have tried and it worked] So final version should be this
String firstName = console.readLine(); String lastName = console.readLine();
console.printf("First name: %s", firstName);
This should fix your code. Make sure to understand the code fully that I and @Tabatha Trahan adviced.