Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial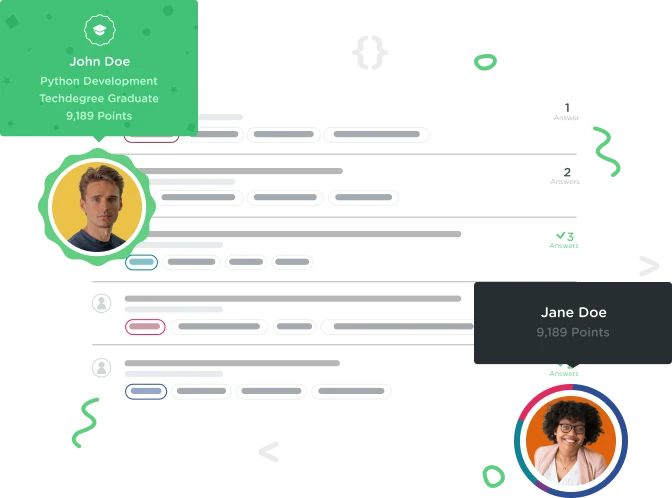

Yiu Ming Lai
5,861 PointsDid you instantiate a new JsonSerializer?
What is wrong with my code? I couldnt find out what is the problem, Please Help!
using System;
using System.IO;
using System.Collections.Generic;
using Newtonsoft.Json;
namespace Treehouse.CodeChallenges
{
public class Program
{
public static void Main(string[] arg)
{
}
public static List<WeatherForecast>DeserializeWeather(string fileName)
{
var weatherForecasts = new List<WeatherForecast>( );
var serializer = new JsonSerializer (fileName );
using (var reader = new StreamReader(fileName))
using (var jsonReader = new JsonTextReader(reader))
{
using (var jsonReader = new JsonTextReader(reader))
weatherForecasts = serializer.Deserialize<List<WeatherForecast>>(jsonReader);
}
return weatherForecasts;
}
public static WeatherForecast ParseWeatherForecast(string[] values)
{
var weatherForecast = new WeatherForecast();
weatherForecast.WeatherStationId = values[0];
DateTime timeOfDay;
if (DateTime.TryParse(values[1], out timeOfDay))
{
weatherForecast.TimeOfDay = timeOfDay;
}
Condition condition;
if (Enum.TryParse(values[2], out condition))
{
weatherForecast.Condition = condition;
}
int temperature;
if (int.TryParse(values[3], out temperature))
{
weatherForecast.Temperature = temperature;
}
double precipitation;
if (double.TryParse(values[4], out precipitation))
{
weatherForecast.PrecipitationChance = precipitation;
}
if (double.TryParse(values[5], out precipitation))
{
weatherForecast.PrecipitationAmount = precipitation;
}
return weatherForecast;
}
}
}
using System;
/* Sample JSON
[
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 0:00",
"condition": "Rain",
"temperature": 53,
"precipitation_chance": 0.3,
"precipitation_amount": 0.03
},
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 6:00",
"condition": "Cloudy",
"temperature": 56,
"precipitation_chance": 0.08,
"precipitation_amount": 0.01
},
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 12:00",
"condition": "PartlyCloudy",
"temperature": 70,
"precipitation_chance": 0,
"precipitation_amount": 0
},
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 18:00",
"condition": "Sunny",
"temperature": 76,
"precipitation_chance": 0,
"precipitation_amount": 0
},
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 19:00",
"condition": "Clear",
"temperature": 74,
"precipitation_chance": 0,
"precipitation_amount": 0
}
]
*/
namespace Treehouse.CodeChallenges
{
public class WeatherForecast
{
public string WeatherStationId { get; set; }
public DateTime TimeOfDay { get; set; }
public Condition Condition { get; set; }
public int Temperature { get; set; }
public double PrecipitationChance { get; set; }
public double PrecipitationAmount { get; set; }
}
public enum Condition
{
Rain,
Cloudy,
PartlyCloudy,
PartlySunny,
Sunny,
Clear
}
}
2 Answers

Yiu Ming Lai
5,861 PointsWhat is the problem with this code? it is still not working. I already added the Newtonsoft.Json namespace
public static List<WeatherForecast> DeserializeWeather(string fileName)
{
var weatherForecasts = new List<WeatherForecast>( );
using (var reader = new StreamReader(fileName))
using (var jsonReader = new JsonTextReader(reader))
{
var serializer = new JsonSerializer( )
weatherForecasts = serializer.Deserialize<List<WeatherForecast>>(jsonReader);
}
return weatherForecasts;
}
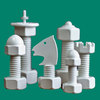
Steven Parker
241,809 PointsIt looks like you're on task 4 but you did not complete task 3 yet.
In particular, you overlooked the instruction to: "Add a using directive for the Newtonsoft.Json namespace. "
Then for task 4, it looks like you missed this instruction: "Inside the using block, instantiate a new JsonSerializer named serializer." Instead, it appears that you copied your line that creates jsonReader and put the copy inside the block. Unintended copy/paste, perhaps?

Yiu Ming Lai
5,861 PointsI tried, but is still saying "Did you instantiate a new JsonSerializer?"