Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial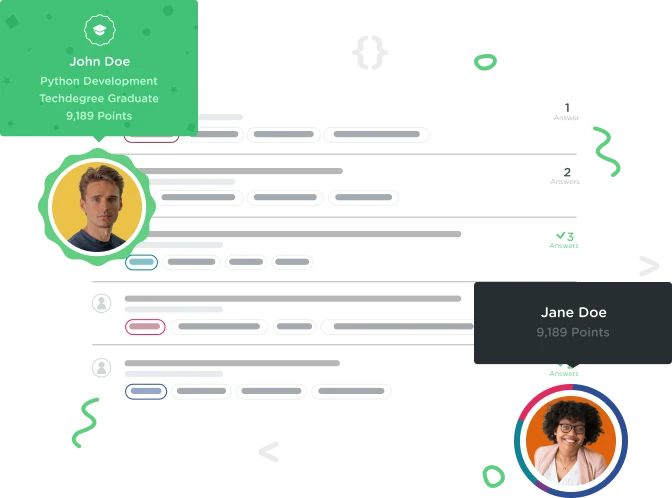

Oraseanu Daniel
1,278 PointsDid you remember to call the assist method on the CustomerSupportRep? I actualy did.
This challenge seems pretty hard to me.. maybe someone can help:
- I don't understand why I get this error since I did call the assist method.
- It seems to me a little strange that while loop because I don't have control over the Queue. This can be an infinite loop unless you guys do something in the back and populate the queue. Else the requirement is wrong - I should be asked to add elements in the queue.
- I suppose I can call the CustomerSupportRep constructor with any name I like, right?
import java.util.ArrayDeque;
import java.util.Queue;
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr;
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
while (mSupportReps.isEmpty()) {
playHoldMusic();
}
csr = new CustomerSupportRep("csrNameHere");
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
csr.getAssistedCustomers().add(customer);
csr.assist(customer);
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
mSupportReps.add(csr);
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
import java.util.List;
import java.util.ArrayList;
public class CustomerSupportRep {
private String mName;
private List<Customer> mAssistedCustomers;
public CustomerSupportRep(String name) {
mName = name;
mAssistedCustomers = new ArrayList<Customer>();
}
public void assist(Customer customer) {
System.out.printf("Hello %s, my name is %s, how can I assist you.%n",
customer.getName(),
mName);
System.out.println("...");
System.out.println("Is there anything else I can help you with?");
mAssistedCustomers.add(customer);
}
public List<Customer> getAssistedCustomers() {
return mAssistedCustomers;
}
}
public class Customer {
private String mName;
public Customer(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
2 Answers

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsHey Oraseanu,
I thought a WHILE loop was a little awkward, too, so I just used an IF ELSE block.
You don't need to instantiate a new CustomerSupportRep object since those objects already exist in the mSupportRep queue. So, you just need to pull a CustomerSupportRep object out of the queue and assign it to the csr variable, like so:
csr = mSupportReps.poll();
You don't need this line:
csr.getAssistedCustomers().add(customer);
Because the Customer that you pass to the assist() method is added to the CustomerSupportRep's mAssistedCustomers field at the end of the assist() method (see last line in assist() method below):
public void assist(Customer customer) {
System.out.printf("Hello %s, my name is %s, how can I assist you.%n",
customer.getName(),
mName);
System.out.println("...");
System.out.println("Is there anything else I can help you with?");
mAssistedCustomers.add(customer);
}
Let me know if you have any more questions on this.

Oraseanu Daniel
1,278 Pointsit worked, thanks! This solution is not as expected in the notes: "Wait until there is an available rep in the queue. HINT: That while assignmentcheck loop syntax we used to read files seems pretty similar" but it worked!
Maybe the notes are not always the best.