Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial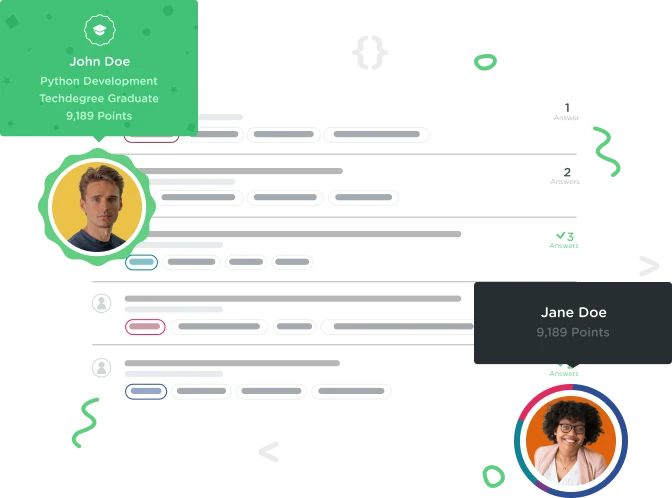
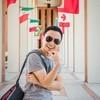
hamsternation
26,616 PointsDidn't find the right directory for year-month-day date?
The prompt: Create a function named create_daily_dir in backup.py. This function should take a string which will be a date in either year-month-day (2012-12-22) or month-day-year (12-22-2012) format. Use that to create a directory like 2012-12-22 (year-month-day) in the financial directory (which is in the current directory).
This means that by calling create_daily_dir("04-22-2017"), we'd have a directory structure like financial/2017-04-22/.
My code:
import os
def create_daily_dir(string):
if int(string.split("-")[2]) > 31:
new_str = string.split("-")[2] + '-' + string.split("-")[0] + '-' + string.split("-")[1]
else:
new_str = string
os.makedirs(new_str, exist_ok=True)
I tried this out on my system and it creates the folder just like the prompt asks, am I missing something?
Is there a better way to parse datetime (datetime.strptime
?) that recognizes whether the string is YYYYMMDD or MMDDYYYY without an if statement?
3 Answers
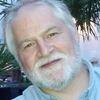
Jeff Muday
Treehouse Moderator 28,722 PointsYou're incredibly clever! You will make a good programmer! The only thing you missed to pass the challenge was adding 'financial/' as the beginning part of the path, see below.
import os
def create_daily_dir(string):
if int(string.split("-")[2]) > 31:
new_str = string.split("-")[2] + '-' + string.split("-")[0] + '-' + string.split("-")[1]
else:
new_str = string
os.makedirs('financial/' + new_str, exist_ok=True)
Another way this can be done is to import some additional functionality from the datetime library. My version will throw an error if a date comes in that does not conform to the format. Is that better? You can be the judge.
import os
import datetime
def create_daily_dir(string, path='financial'):
# try to decode date string to a datetime
try:
this_date = datetime.datetime.strptime( string, "%Y-%m-%d" ) #format type YYYY-mm-dd
except:
this_date = datetime.datetime.strptime( string, "%m-%d-%Y" ) #format type mm-dd-YYYY
# convert back to the proper format
date_str = datetime.datetime.strftime(this_date, '%Y-%m-%d')
os.makedirs(os.path.join(path, date_str))
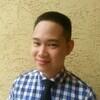
Tom Nguyen
33,501 PointsWhat I did
import os
def create_daily_dir(string):
date = string.split("-")
if len(date) == 4: # the format coming in is mm-dd-yyyy
directory_path = "financial/" + "{}-{}-{}".format(date[2],date[0],date[1])
os.makedirs(directory_path, exist_ok=True)
else: # the format of string is yyyy-dd-mm
os.makedirs("financial/"+string, exist_ok=True)
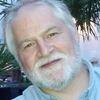
Jeff Muday
Treehouse Moderator 28,722 PointsDateutil is very cool (and simpler)! Is it available in our Challenges environment?
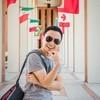
hamsternation
26,616 PointsIt imported successfully :) :D
hamsternation
26,616 Pointshamsternation
26,616 Pointsnice! that's so weird! I could have swore i tried adding the path as well, must have made a typo.
I actually figured out how to solve it with 2 lines! I was out of ideas and found a really useful library, dateutil:
Thanks!