Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial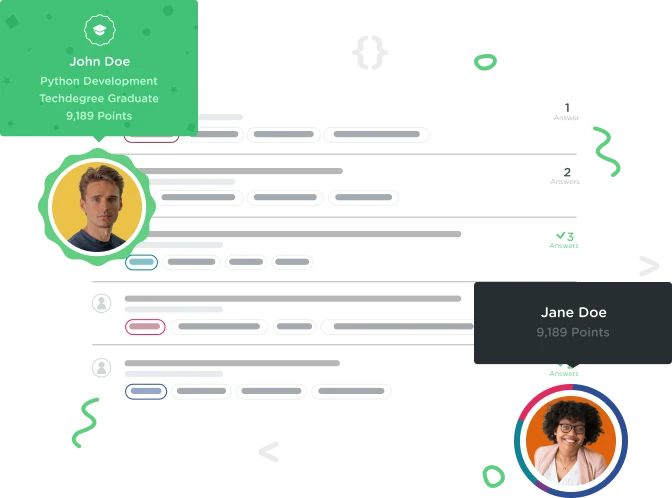

swiftfun
4,039 PointsDidnt fully understand when do we use unary operator (in real cases) as well did not understand why prefix or postfix
Can someone please help?
2 Answers
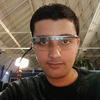
ankushdharkar
7,530 PointsThink of unary operators as a shorthand way of writing code.
Let's say that you have a website, and you are keeping track of how many users visited it. You want to send out an email to yourself when you hit a 1000 views. Without unary operations, you would write something like
webpageViews=webpageViews+1
if(webpageViews == 1000){
//send email
}
Using the unary operator, my code becomes more concise (and with time, more readable)
webpageViews++
if(webpageViews == 1000){
//send email
}
I can still reduce the lines by doing a postfix increment (after that instruction in which the unary operator is used)
if(webpageViews++ == 1000){
//send email //WAIT! Houston, we have a problem
}
In the above code, the value of webpageViews is incremented AFTER the value of the variable is fetched. So, first the value is returned to the expression, and then it is incremented. That means, on the 1000th user visiting your page, the current value of webpageViews i.e 999 is compared against 1000 first. Since it's not equal, the email is not sent. And then, the webpageViews increases to 1000. That means, on the 1001th user, you will get an email, but that is not what you wanted.
Basically, the wrong code equates to
if(webpageViews == 1000){
//send email
}
webpageViews = webpageViews+1
We wanted the value of webpageView to increment first, and this fresh value to be compared against 1000. So that, at the 1000th user, we get our email. For this, I will use a prefix operator, i.e increment first, then use in the operation
if( ++webpageViews == 1000){
//send email
}
This way, we get the job done in a more concise way. Also, the unary operators have their performance benefits in the system, which you don't need to get into (and may never need to)
I hope this helps you out.
Best

swiftfun
4,039 PointsAs well, he mentioned that levelScore-- would decrement by one. How about if we want to decrement by 2, or 3?
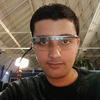
ankushdharkar
7,530 PointsYou can do a
levelScore -= 2
or the usual
levelScore = levelScore - 2