Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial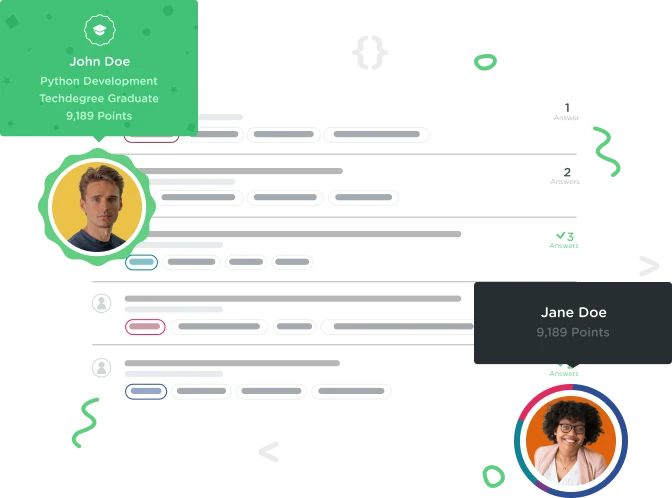
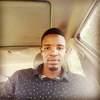
Savious Munyaradzi Ngundu
16,920 PointsDidn't get back a properly hashed password; Couldn't create a user
please help me out been stuck for days now
import datetime
from argon2 import PasswordHasher
from itsdangerous import (TimedJSONWebSignatureSerializer as Serializer,
BadSignature, SignatureExpired)
from peewee import *
DATABASE = SqliteDatabase('courses.sqlite')
HASHER = PasswordHasher()
class User(Model):
username = CharField(unique=True)
email = CharField(unique=True)
password = CharField()
class Meta:
database = DATABASE
@classmethod
def create_user(cls, username, email, password, **kwargs):
email = email.lower()
try:
cls.select().where(
(cls.email == email) | (cls.username**username)
).get()
except cls.DoesNotExist:
user = cls(username=username, email=email)
user.password = user.set_password(password)
cls.create(email=email, password=generate_password_hash(password))
user.save()
return user
else:
raise Exception("User with that email or username already exists.")
@staticmethod
def set_password(password):
return HASHER.hash(password)
@staticmethod
def hash_password(password):
return HASHER.hash(password)
def verify_password(self, password):
return HASHER.verify(self.password, password)
def generate_auth_token(self, expires=3600):
serializer = Serializer(config.SECRET_KEY, expires_in=expires)
return serializer.dumps({'id': self.id})
@staticmethod
def verify_auth_token(token):
serializer = Serializer(config.SECRET_KEY)
try:
data = serializer.loads(token)
except (SignatureExpired, BadSignature):
return None
else:
user = User.get(User.id == data['id'])
return user
class Course(Model):
title = CharField()
url = CharField(unique=True)
created_at = DateTimeField(default=datetime.datetime.now)
class Meta:
database = DATABASE
class Review(Model):
course = ForeignKeyField(Course, related_name='review_set')
rating = IntegerField()
comment = TextField(default='')
created_at = DateTimeField(default=datetime.datetime.now)
created_by = ForeignKeyField(User, related_name='review_set')
class Meta:
database = DATABASE
def initialize():
DATABASE.connect()
DATABASE.create_tables([User, Course, Review], safe=True)
DATABASE.close()
3 Answers
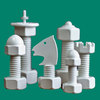
Steven Parker
230,274 PointsThis code looks like it came from a different challenge than the one linked by the button. The button challenge code works with a database of recipes and has methods associated with that, but this code seems to be working on a database of courses and has entirely different methods. The task objectives are obviously very different as well. This code clearly will not work for that challenge.
Starting the challenge over is probably the only way to deal with a snafu of this magnitude.
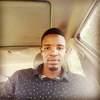
Savious Munyaradzi Ngundu
16,920 Pointshonestly i am stuck can you assist me
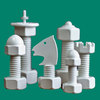
Steven Parker
230,274 PointsYes, but only by pointing out that you need to start the challenge over, use the code the challenge gives you, and add only what the instructions ask for.
The code you have above does not go with the challenge and it's not possible to "fix" it to work.
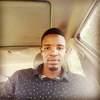
Savious Munyaradzi Ngundu
16,920 Pointsi got right thanks
Savious Munyaradzi Ngundu
16,920 PointsSavious Munyaradzi Ngundu
16,920 Pointsnot really but i am stuck for days now failing to work it out, please help me out
Savious Munyaradzi Ngundu
16,920 PointsSavious Munyaradzi Ngundu
16,920 Pointsplease help me
Steven Parker
230,274 PointsSteven Parker
230,274 PointsAs I was trying to explain, this code does not go with the challenge. It seems to be from a different challenge, or maybe from the video follow-along. It is not possible to pass this challenge using this code.
You will need to start the challenge over, and be sure you do not paste any code from your video follow-along project into the challenge. Start with the code the challenge gives you, and add only what the instructions ask for.