Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial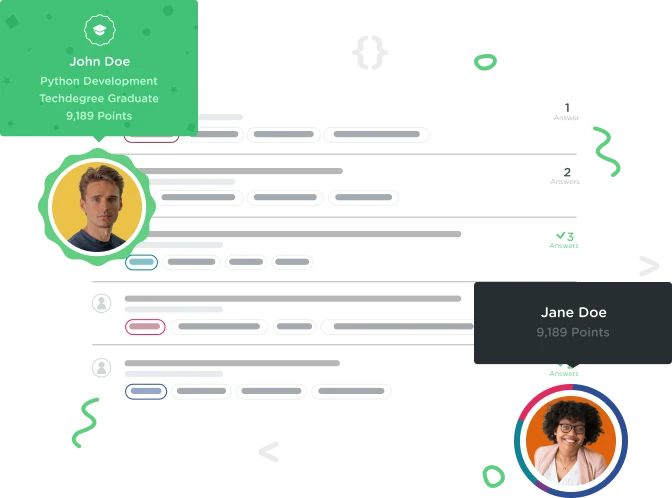
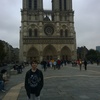
João Sulzbach
2,611 PointsDidn't understand how to use the "enumerate" function to handle this combo challenge. Can someone show me how to solve?
I would really aprecciate if someone could explain (detailed, if it's not too much to ask) how to use enumerate, specially with this challenge.
Challenge: Create a function named combo() that takes two iterables and returns a list of tuples. Each tuple should hold the first item in each list, then the second set, then the third, and so on. Assume the iterables will be the same length.
1 Answer
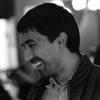
Martin Cornejo Saavedra
18,132 Points#Solution with enumerate:
def combo(iter_1, iter_2):
my_list = [] #our empty list to fill
for idx,data in enumerate(iter_1): #doesn't matter if
#iter_1 or iter_2
my_tuple = (iter_1[idx], iter_2[idx])
my_list.append(my_tuple)
return my_list
#=======================================================================
#Solution without enumerate:
def combo(iter_1, iter_2):
length = len(iter_1) #both iterables are the same length
#it doesn't matter if its list 1 or 2
my_list = [] #let's create our list to fill
for index in range(length):
my_tuple = (iter_1[index], iter_2[index])
my_list.append(my_tuple)
return my_list
#========================================================================
#Solution with built-in function zip():
def combo(iter_1, iter_2):
return list(zip(iter_1, iter_2))
João Sulzbach
2,611 PointsJoão Sulzbach
2,611 PointsThank you! Let me see if I understood the solution with enumerate correctly: for each index of iterable 1 or iterable 2, cause, how you said it, it doesn't matter which of them (is that because they're the same length?) Python will create a new tuple containing the index of each iterable. Am I correct? I think the solution without enumerate helped me a lot. Once again, thank you!