Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial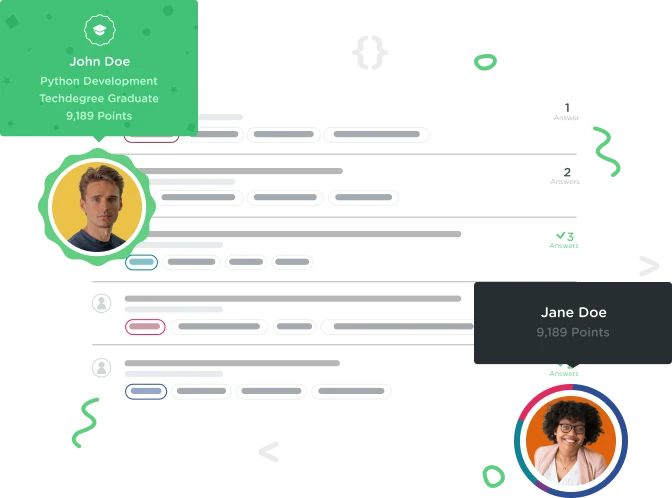

jafararifdjanov
1,215 Pointsdidn't understand on how to prompt kwargs
How to do it?
1 Answer
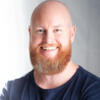
Mark Ryan
Python Web Development Techdegree Graduate 28,648 PointsHi, Jafar!
When we want to make an instance of a Class in Python, we can provide arguments to its init method.
class RaceCar:
def __init__(self, color, fuel_remaining):
self.color = color
self.fuel_remaining = fuel_remaining
racecar = RaceCar("blue", 68)
We can also provide keyword arguments to the init method.
class RaceCar:
def __init__(self, color, fuel_remaining, doors=None):
self.color = color
self.fuel_remaining = fuel_remaining
self.doors = doors
no_doors_racecar = RaceCar("red", 44)
Above we're saying that our RaceCar instance has no doors by default. What if we want one of our RaceCar instances to have two doors?
class RaceCar:
def __init__(self, color, fuel_remaining, doors=None):
self.color = color
self.fuel_remaining = fuel_remaining
self.doors = doors
two_door_racecar = RaceCar("red", 44, doors=2)
What if our car may have many keyword arguments that we know about? That's where **kwargs and kwargs.get() comes in.
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
self.doors = kwargs.get('doors')
self.seats = kwargs.get('seats')
self.lights = kwargs.get('lights')
racecar_with_doors_lights_seats = RaceCar("red", 44, doors=2, seats=4, lights=4)
But what if we don't know the characteristics of a car we want to make in the future? What if we want to be flexible with keyword arguments - accept any number of keyword arguments?
class RaceCar:
def __init__(self, color, fuel_remaining, **kwargs):
self.color = color
self.fuel_remaining = fuel_remaining
for key, value in kwargs.items():
setattr(self, key, value)
ferrari_racecar = RaceCar("red", 44, doors=2, seats=4, lights=4, make="Ferarri", model="F40")
Above allows our RaceCar class to accept any number of keyword arguments. The kwargs.items() will reveal each keyword argument and its corresponding value. The setattr() will set each keyword argument and its value to your instance. Now your class is more flexible!
I hope this helps!