Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial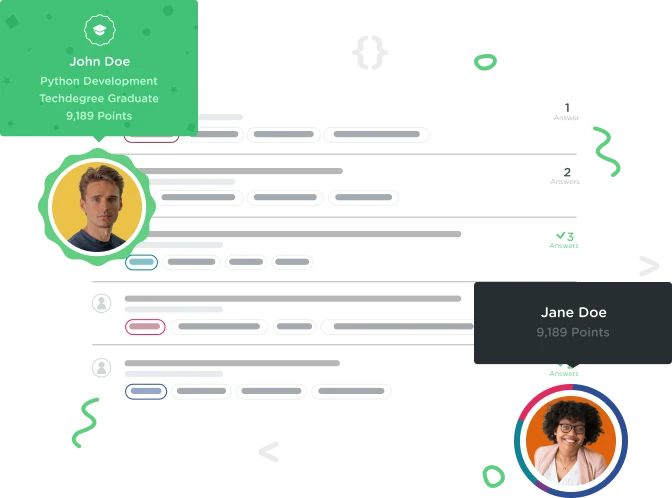

Dani G
Courses Plus Student 229 PointsDifference between a method and a Computed Property?
So, if we could return a value using both a method and a computed property, what's the difference between them (besides that one is a method, the other one is not) and which one should we use in what particular cases?
2 Answers

Greg Kaleka
39,021 PointsMethods are things the object can do, while properties are things about the object. A computed property is just a way to make it easier to get to an attribute of an object.
For example, imagine you had a Person
class, which had a yearOfBirth
property. You want to figure out how old the person is. Do you use a method to calculate it or set a calculated property?
Well, we're trying to figure out someone's age
, so I think it makes the most sense to set a calculated property. We could do this easily, and refer to dani.age
later on to get your age.
Here's an example:
class Person {
let name: String
let yearOfBirth: Int
var age: Int {
return 2015 - yearOfBirth // note, this is simplified. I would probably use NSDate instead to really calculate the age, and have it update itself as time went on
}
init(name: String, yearOfBirth: Int){
self.name = name
self.yearOfBirth= yearOfBirth
}
}
let dani = Person(name: "Dani", yearOfBirth: 1900)
dani.name // "Dani"
dani.yearOfBirth // 1900
dani.age // 115
Gosh, you're old! ;-)
Make sense?

Dani G
Courses Plus Student 229 PointsYes, it does make sense! Thanks!