Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial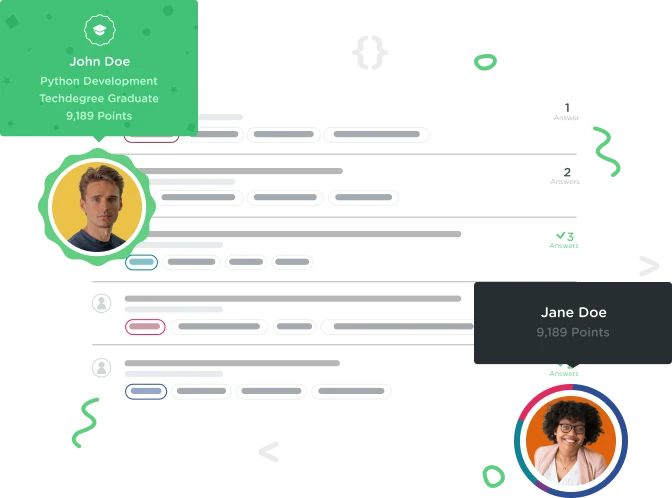
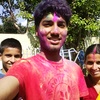
Naivedya Bansal
5,483 Pointsdifference between == and ===
what is the difference between equal to and strictly equal to?
4 Answers

Cristian Altin
12,165 PointsJavascript makes a lot of things easier for you so check out this example I made to help you understand. Consider these two variables.
a = "2";
b = 2;
Now we will begin testing:
//Value equality testing against number 2
a == 2;
> true
b == 2;
> true
//Value equality testing against string "2"
a == "2";
> true
b == "2";
> true
As you can see a == 2 outputs true even if variable a is a string containing a number and I'm testing it against the number 2. This is because "==" tests value, not type. They are all true here unless I try a == 3 or anything else which returns false. Next you will see how "===" equality works differently instead.
//Full equality testing against number 2
a === 2;
> false
b === 2;
> true
//Full equality testing against string "2"
a === "2";
> true
b === "2";
> false
Now you can see that with "===" value AND type are needed to pass the test.

Conor Graham
7,956 Points" ===" or strict comparison means that it has the same type and value. "==" just checks the value. Its better to use "===" and check the type, and if anything breaks you can just convert it from Type A to Type B
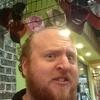
Richard Skinner
5,839 PointsJust to add to the already great answers here.
The '==' operator compares two values without consideration to the type (string, number, etc). This is why '3' == 3 is considered true to the '==' operator.
The '===' operator compares two values and returns true if and only if the value and type are the same. Basically, both values have to be the same in every way for the strict equal to operator to return true.

Nguyen Quach
1,827 PointsWhat is a real world scenario in which we would apply this? In Java, were not using "===" so I am curious as to what benefits this yields?

Damian Padilla
6,107 Points"==" is a comparison that does not consider type while "===" is a strict comparison that does consider type.
"===" Both the type and value have to be the same. Ex: 4 === "4" //would print false since the type and value are not the same
"==" Ex: 4=="4" //would print true.
MURAT AYDIN
2,942 PointsMURAT AYDIN
2,942 PointsThanks for answer.
ammonquackenbush
3,867 Pointsammonquackenbush
3,867 PointsGood answer.