Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial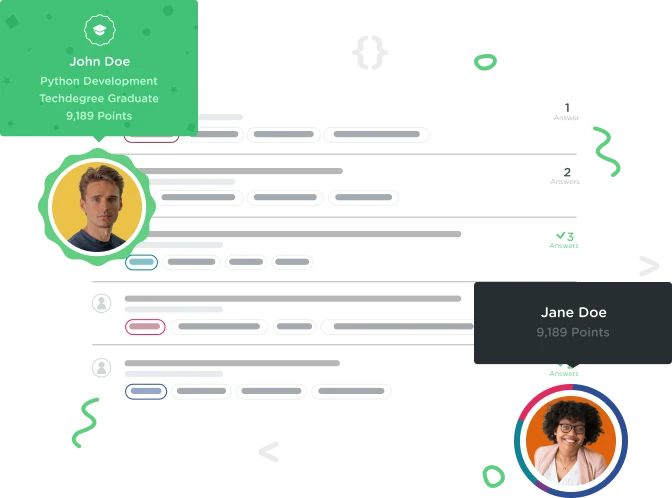

Haoz Bach Ly
3,709 PointsDifference between appendChild and append Using innerHTML
I have two issues. First is about innerHTML property:
Why this method's result is only 'name' in the console instead of '<li>name</li>':
document.createElement('li').innerHTML = 'name';
After searching in the internet, the correct method for the result '<li>name</li>':
var name = document.createElement('li');
var add = document.createTextNode('name')
name.appendChild('add');
In addition to that,I am not sure the how the append and appendChild work in the add() function below:
This function adds more items to the ul list.
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<button>Hide</button>
<div id='event_1'>
<p class='event_2'>Things that are purple:</p>
<input type='text' class='event_2'>
<button class='event_2'>Change the description</button>
<ul class='addList'>
<li>grapes</li>
<li>amethyst</li>
<li>lavender</li>
<li>plums</li>
</ul>
<input type='text' class='addList'>
<button class='addList'>List</button>
</div>
<script src="app.js"></script>
</body>
</html>
let list = document.querySelector('ul.addList');
const addButton = document.querySelector('button.addList');
var x = [];
var z;
function add () {
z = document.createTextNode(document.querySelector('input.addList').value);
x.push(document.createElement('li'));
x[x.length - 1].appendChild(z);
list.append(x[x.length-1]);
}
addButton.addEventListener('click', add);
1 Answer

Neil McPartlin
14,662 PointsHi Haoz.
To avoid confusion, just temporarily 'comment out' your app.js entry in your index.html file. Now in your browser, view index.html and open your Javascript console.
Issue 1: Your single line code is fine (as far as it goes). In one line, you have created the <li></li> tags AND assigned the text 'name' inside them. It is just the assigment part that you see confirmed in your console. But right now, you have no label for which you can search, to see that you have been successful. So break down your code like so and type or copy/paste line by line into your console...
var listItem = document.createElement('li') // this just creates the tags.
listItem // <li></li> - To confirm this is true.
listItem.innerHTML = 'name' // 'name' - So this assigns the text 'name' inside the already existing tags, but will not show you those tags. (Instead of innerHTML you could use innerText here too).
listItem // <li>name</li> Now you can see your hoped for response.
So far so good. So now let's actually add this new entry to the page html (i.e. add it to the DOM).
// First we select and give a name to the current list
let list = document.querySelector('ul.addList')
// Finally we add your new entry.
list.appendChild(listItem) // <li>name</li> - So in the console, the new entry is confirmed in full, plus in your main html you can see the 5th entry is now on view too.
Issue 2: First thing to mention is that where you see append(), appendChild() will work there too. Most Google searches seemed to suggest that append() is a JQuery command, but I just found this which suggests it is also a command in native JavaScript which is designated as 'experimental technology'.
https://developer.mozilla.org/en-US/docs/Web/API/ParentNode/append
As for the function, it seems that it creates an array 'x' which retains a list of what will be the 5th and subsequent items that are being added. (The first 4 already present in the index.html). So z is the 'text-node' which is the text that was typed in before the button was pushed.
x.push just adds the li tags to the array. Then ....appendChild(z) is adding the 'text-node' as a child to the parent 'li' elements. Finally list.appendChild(.... is adding the combined li tags + text to the bottom of the list.