Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial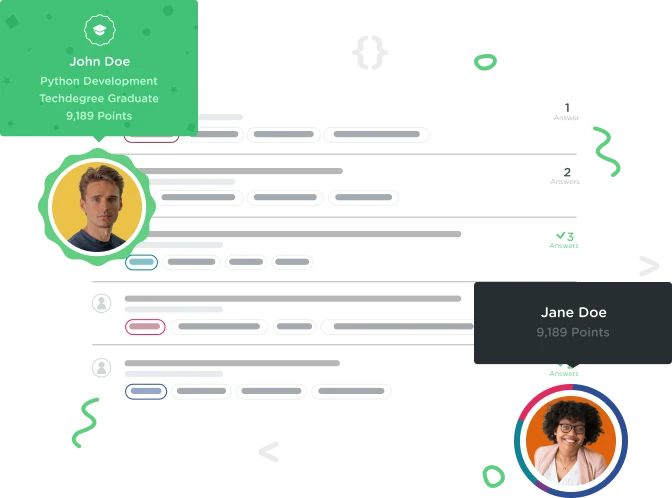

Carlos Enrique Castañeda Gutiérrez
13,886 PointsDifference between "attr()" and "prop()", am I right ?
Hello:
Can someone explain me the difference between an attribute and property with jQuery
I have guess, I'm not sure if is ok:
- Attribute: Is something that can have a complex value as "src", "href".
- Property: is something that can have few fixed values as "selected".
Am I right?
Thanks
4 Answers

alex novickis
34,894 Pointsfirst we need to think about what is an attribute and what is a property -
Attributes provide additional info about an HTML element - for example <input id="some_id" value="some_value"> basically they are things with name="value" in HTML constructs the point though is we are now talking about what is contained in the original HTML file
Now once the HTML is loaded and placed into the DOM HTML structure, then it becomes a node in that tree and it becomes a property of that node.
Content wise those things are the same, except if you modify
so now we can discuss jQuery.attr() vs jQuery.prop()
the jQuery.attr() will give the value of the first matching attribute in the original HTML file, while
jQuery.prop() will give the value of that same object but instead fetches it from the populated DOM structure
in many cases the returned item will be the same - but keep in mind one is the current state vs the original state
But - in earlier versions of jQuery (before 1.6) there are some special considerations, so if that is what you are doing then I would take a closer read in api.jquery.com/prop
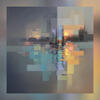
Montazar Al-Jaber
19,611 Points"Generally, DOM attributes represent the state of DOM information as retrieved from the document, such as value attribute in the markup <input type='text' value='abc'>. DOM properties represent the dynamic state of the document: for example if the user clicks in the input element above and types def the .prop('value') is abcdef but the .attr('value') remains abc." - jQuery 1.6 changelog.
You can try it out yourself:
<form>
<input type='text' value='abc'>
<button id='go'>Go</button>
</form>
Begin by clicking the 'Go' button before you type anything. You'll see the current property value and the attribute value (defined in the document). Now, type something into the field, and hit the 'Go' button.
$('#go').click(function() {
var attrMethod = $('input[type="text"]').attr('value');
alert(attrMethod);
var propMethod = $('input[type="text"]').prop('value');
alert(propMethod);
});
The difference is only remarkable while retrieving an attribute's value.
On the other hand, setting a value with either of the methods do not make any difference, except that the .prop() method is tad bit faster. As a matter of fact, the attr() method internally calls the .prop() method to make the change.
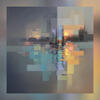
Montazar Al-Jaber
19,611 Points"Generally, DOM attributes represent the state of DOM information as retrieved from the document, such as value attribute in the markup <input type='text' value='abc'>. DOM properties represent the dynamic state of the document: for example if the user clicks in the input element above and types def the .prop('value') is abcdef but the .attr('value') remains abc." - jQuery 1.6 changelog.
You can try it out yourself:
<form>
<input type='text' value='abc'>
<button id='go'>Go</button>
</form>
Begin by clicking the 'Go' button before you type anything. You'll see the current property value and the attribute value (defined in the document). Now, type something into the field, and hit the 'Go' button.
$('#go').click(function() {
var attrMethod = $('input[type="text"]').attr('value');
alert(attrMethod);
var propMethod = $('input[type="text"]').prop('value');
alert(propMethod);
});
The difference is only remarkable while retrieving an attribute's value.
On the other hand, setting a value with either of the methods do not make any difference, except that the .prop() method is tad bit faster. As a matter of fact, the attr() method internally calls the .prop() method to make the change.
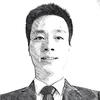
Hanxiao Jiang
8,526 PointsI searched, finding that .attr() only returns String while .prop() return other types of value. For example, after jQuery 1.6, .attr() will return a string "selected" , "checked" for
selected, checked
attributes.
While .prop will return "true" for
selected, checked
attributes