Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial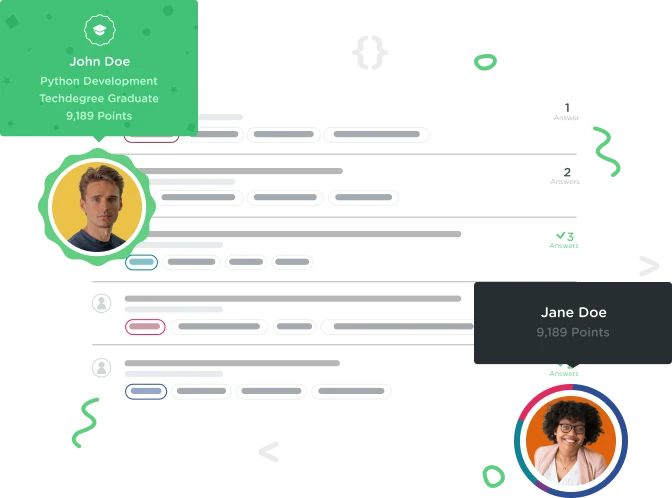

Rachel Lim
720 Pointsdifference between do while and while loop using secret password challenge tasks
In this challenge, "A Closer Look at Loop Conditions," this is correct:
var secret = prompt("What is the secret password?");
while (secret !== "sesame") {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
but in this challenge, "Create a do...while loop," this is correct:
var secret;
do {
secret = prompt("What is the secret password?");
}
while ( secret !== "sesame" )
document.write("You know the secret password. Welcome.");
Why is it in the first challenge the var secret is declared outside the function and then re-declared inside the function but in the second challenge this isn't so?
2 Answers
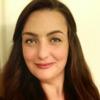
Jennifer Nordell
Treehouse TeacherHi there! In reality, in both cases the secret
variable is only declared once. The var
part is the declaration. The difference between the do while loop and the while loop is that a do while
is always guaranteed to run at least once. This is not the case with the while
.
In the while, we evaluate if the word entered was equal to "sesame" . This means that we have to have gotten a guess from them before the evaluation. If it was, then that loop never runs and we skip directly to the print out of acceptance of the secret word. But if it wasn't, then we continue to ask over and over for the secret word until they get it.
In the do while loop, because it's guaranteed to run at least once, we use that to get the user's guess then we evaluate if they got it correct. If they did, we print out the acceptance of the word, otherwise we continue to prompt the user.
Hope this helps!
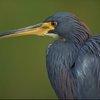
Matthew Garza
26,312 Pointsvar secret = "Matthew";
do { secret = prompt("What is the password?!"); } while (secret !== "Matthew") { document.write("Good job, you guessed it right"); }
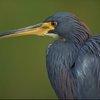
Rachel Lim
720 PointsRachel Lim
720 PointsSo in do while loop because its needs to run first, the variable will always be assigned to a value or string inside the function. However, in a while loop, we need to evaluate the condition first, so the variable will be assigned to a value or string outside of the function. Correct?
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherRachel Lim the while loop doesn't necessarily need to run first. But we've chosen to let the user guess once and then evaluate if they were correct. We could start it with a true evaluation by doing this:
This would cause the evaluation to be true and begin the while loop, and we'll continually get the user's guess.
But if we did this in the do while loop:
This code would ask the user for the password. But then it wouldn't evaluate if it's correct or not. It'd just immediately ask the user again. But because it's only ever evaluated in the do while loop, they have no way to know if what they typed in first was correct. So, if they managed to guess the correct password the first time, they'd never know.
Rachel Lim
720 PointsRachel Lim
720 PointsThank you! It makes sense. It seems simple, don't know why I couldn't wrap my head around it. But thank you