Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial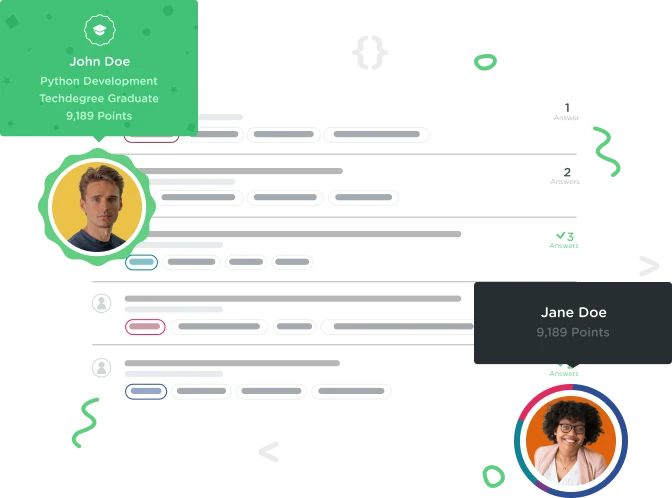

Stayl T
968 PointsDifference between implementing and importing?
What is the purpose of using 'implements' on 'Comparable' and then using its compareTo() method? Can't we just import the class 'Comparable' and use the method as @override? We are using @override anyway!
1 Answer

Derek Markman
16,291 PointsI'm not sure if you already know this, but, Comparable is an interface, and not a Class.
You can both implement and import an interface. If you import an interface you don't have to implement it. But, if you implement an interface , you do have to import it if the interface is declared in a different package than the class that implements it.
Basically, what an import statement does, is it tells that file(package) what classes/interfaces are available to be used. But say, you had a main class that implements/imported the Comparable interface, if you were to make a class that extends your main class, but in a different package, that sub-class must also import the Comparable interface.
Let me know if this is confusing and I can try to re-explain.
Stayl T
968 PointsStayl T
968 PointsI didn't understand it completely. Can you explain with the help of an example? What is the major difference between an interface and a class?
Thanks.
Derek Markman
16,291 PointsDerek Markman
16,291 PointsSure, here's a code example.
Bounceable.java
Ball.java
SuperBouncyBall.java
Since SuperBouncyBall is extending a class that is implementing an interface, the sub-class(SuperBouncyBall) MUST implement the Bounceable interface's methods.
To fully understand these code examples you need to know that in an interface, all of the methods are public and abstract. I don't wanna go into too much detail with abstract methods/classes, all you need to know is an interface IS ALWAYS abstract, and so are its methods. An abstract method has NO implementation, just the declaration of the method itself, and ANY concrete (non-abstract) class that implements an interface MUST implement all of that interfaces methods.
Technically, the SuperBouncyBall class doesn't need to implement the Bounceable interface's methods, since they have already been inherited by Ball.java.
In Ball.java I could have used the Bounceable interface two different ways:
or
Stayl T
968 PointsStayl T
968 PointsOkay now things are getting clearer! Thanks a lot for your effort!
Derek Markman
16,291 PointsDerek Markman
16,291 PointsNo problem, let me know if you have any other questions.