Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial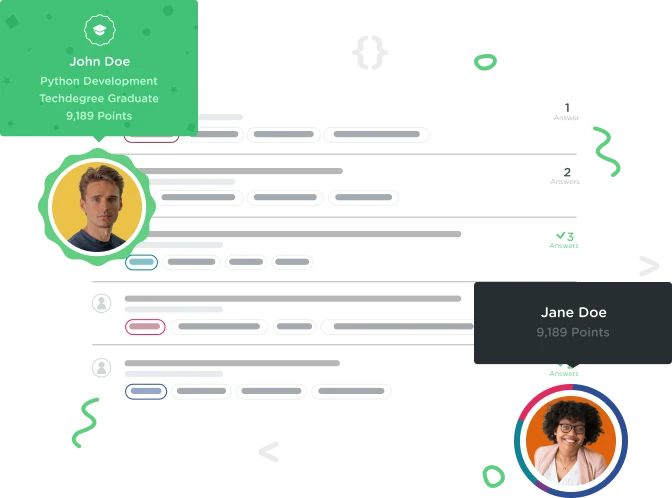

Zach Widhalm
6,406 PointsDifference between intersection and subset?
I know the attached code works with intersection instead of subset, but could someone explain why? Maybe my understanding of the two are incorrect but I figured if the passed in argument was a subset of the values, the list would be appended with the key. Thanks!
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(arg):
list = []
for keys,values in COURSES.items():
if arg.issubset(values):
list.append(keys)
return list
1 Answer

Brandon Spangler
8,756 PointsI think your confusion stems from a lack of understanding the difference between a subset and the intersection of two sets. An intersection between two sets is all the elements the sets have in common. For example, let's say we have two sets, A = {1,2,3,4,5,6,7,8,9} and B = {1,3,5,7,9, 100}. The intersection of these two sets is 1,3,5,7,9. A subset is probably best defined using examples. An example of a subset of set A from above would be {1,2,3} or {1} or {3,7,9} or even {1,2,3,4,5,6,7,8,9}, but set B is NOT a subset of set A because B contains a number that's not in set A. So, the reason intersection works is because we're looking for keys in COURSES whose corresponding set in COURSES.values() has an element that is shared in the set arg. EX: if arg is {"booleans","coffee cup"} then we want our function to return ["Python basics","Java Basics", "PHP basics"] because the intersection between arg and COURSES.values() is {"booleans"}. Now if we had used the subset function, our function would have returned [] because arg is not a subset of any of the sets in COURSES because arg contains an element that is not in the sets in COURSES, namely "coffee cup". However, if arg is just {"booleans"}, then {"booleans"} is the intersection of the two sets and arg is also a subset of some of the sets in COURSES.
I hope that cleared things up a little.
Zach Widhalm
6,406 PointsZach Widhalm
6,406 PointsThanks for taking the time to type all that up! Much appreciated!
Brandon Spangler
8,756 PointsBrandon Spangler
8,756 PointsMy pleasure! Hopefully it helped!