Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial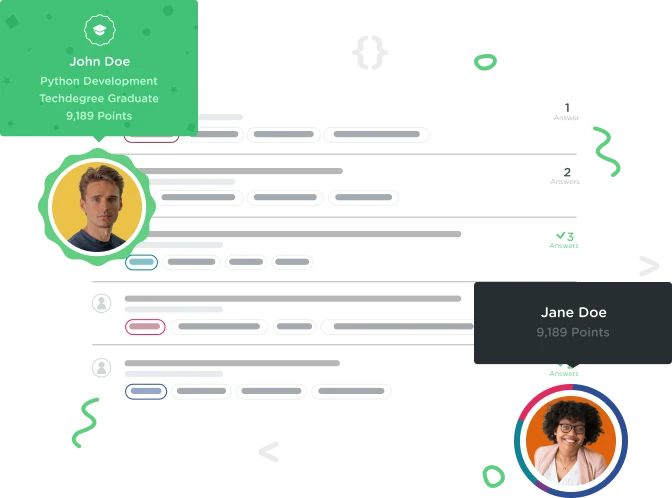

mattlebl
10,849 PointsDifference between setting a variable, and setting a pointer
Functionally, what's the difference? Doug uses the two for his NSNumbers, "mike" and "pi" respectively, and it seems to have no impact on the results.
Thanks!
2 Answers

Stone Preston
42,016 PointsPointers are kind of confusing and many people struggle with understanding them at first, but after a while you start to get it.
pointers are variables that store memory addresses. So all pointers are variables, but not all variables are pointers. its important to note that all objects in objective c are pointers. Because of this, you use them all the time and can pretty much treat them as any other variable. you dont have to dereference (this word is covered more in depth below) them since most methods take pointers to objects as parameters, not the actual objects themselves.
below is a simple example of using pointers with int variables.
int number = 3; //value of number is 3
int *numberPointer = number; //value of numberPointer is the location in memory where number is stored.
in the code above, number is a regular int variable. numberPointer points to number. the value of number is 3. the value of numberPointer is the memory address of number. I could assign the value of numberPointer to another pointer variable like so:
int *anotherPointer = numberPointer; //anotherPointer now points to the same memory address as numberPointer
notice that the value of numberPointer is the memory address of number, it is NOT the value of number. in order to get at the value of the variable that numberPointer points to, you must dereference the pointer. to dereference it, you just put a * in front of the pointer name.
below I assign the value of the variable that numberPointer points to to a new variable by dereferencing it:
int newNumber = *numberPointer; ///newNumber is assigned the value 3, since thats the value that numberPointer pointed to
the difference between a pointer to an int, and a regular int, is that the int variable actually stores the value of that int, whereas the pointer to the int just stores the memory address where the value is stored. the advantage of pointers is that they are very small and easy to pass around.
say you had an array that contained a million items and you declared that as a regular array, anytime you passed that array around it would have to pass all one million of the items which is huge and time consuming. If you had an array pointer that pointed to an array containing a million items, anytime you passed that pointer around it would just be passing the memory address, which is tiny.
Again, all objects in objective c are pointers, so they are used all the time. they are used so much you dont even have to think about it. you (usually) dont have to worry about ever dereferencing them. the only thing you have to remember is to put a * in front of their name when you first declare them. The important thing to remember is that using pointers makes things easier on the computer. There are many other advantages as well, but thats one of the main ones.

Michael Bowen
770 PointsA variable is stored on the stack while an object, which needs a pointer to reference it, is stored on the heap. The stack is local while the heap is not. A variable is a primitive and is usually smaller than the size of an object. However the size of a pointer is the same size as an int primitive, so it improves performance to pass around the pointers instead of the objects themselves in method calls.
Use a primitive when you don't need any special functionality and want to preserve memory. Use an object when you want the properties or methods that come along with that object's class.
mattlebl
10,849 Pointsmattlebl
10,849 PointsThis is great! Thank you so much.