Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial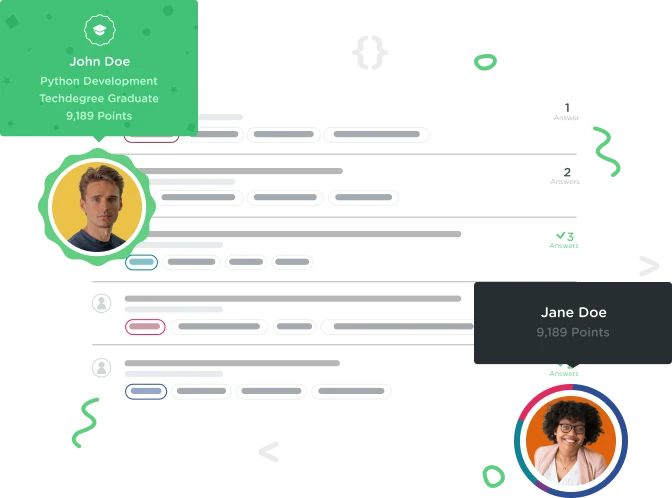

Leigh Maher
21,830 PointsDifference between var and let
Is the difference between var and let, that var is available outside the scope of the block level, but let is only available within that block?
3 Answers

Erik Nuber
20,629 PointsTaken from MDN...
let allows you to declare variables that are limited in scope to the block, statement, or expression on which it is used. This is unlike the var keyword, which defines a variable globally, or locally to an entire function regardless of block scope.
Variables declared by let have as their scope the block in which they are defined, as well as in any contained sub-blocks . In this way, let works very much like var. The main difference is that the scope of a var variable is the entire enclosing function.
function varTest() {
var x = 1;
if (true) {
var x = 2; // same variable!
console.log(x); // 2
}
console.log(x); // 2
}
function letTest() {
let x = 1;
if (true) {
let x = 2; // different variable
console.log(x); // 2
}
console.log(x); // 1
}
My understanding is that var should no longer be used and be replaced with either let or const in E6. The issue with this is that not all browsers are supporting let and const and you have to use a compiler to be safe.
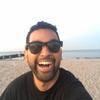
Anthony Albertorio
22,624 PointsHere is a great supplemental video on let, const and template literals that was useful in clearing things up for me: https://www.youtube.com/watch?v=LTbnmiXWs2k&list=PL57atfCFqj2h5fpdZD-doGEIs0NZxeJTX

Leigh Maher
21,830 PointsThat's an excellent resource. Thanks Anthony.

Lars Reimann
11,816 PointsAdding to what Erik wrote, there are also a number of quirks with var. What do you expect to be printed here?
var array = [];
for (var i = 0; i < 6; i++) {
array.push(function () {
return i;
});
}
console.log(array[0]());
Turns out the answer is 6. And it is 6 because variables declared with var don't have block scope. The code is more like this:
var array = [];
var i;
for (i = 0; i < 6; i++) {
array.push(function () {
return i;
});
}
console.log(array[0]());
When we eventually call the functions we pushed into the array, the variable i has the value 6. If we use let instead, we get a new i for every iteration and we get the expected result, i. e. 0:
var array = [];
for (let i = 0; i < 6; i++) {
array.push(function () {
return i;
});
}
console.log(array[0]());
Leigh Maher
21,830 PointsLeigh Maher
21,830 PointsThanks Erik. That's very helpful. I also found this discussion on Stack Exchange which I found quite helpful: http://programmers.stackexchange.com/questions/274342/is-there-any-reason-to-use-the-var-keyword-in-es6