Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial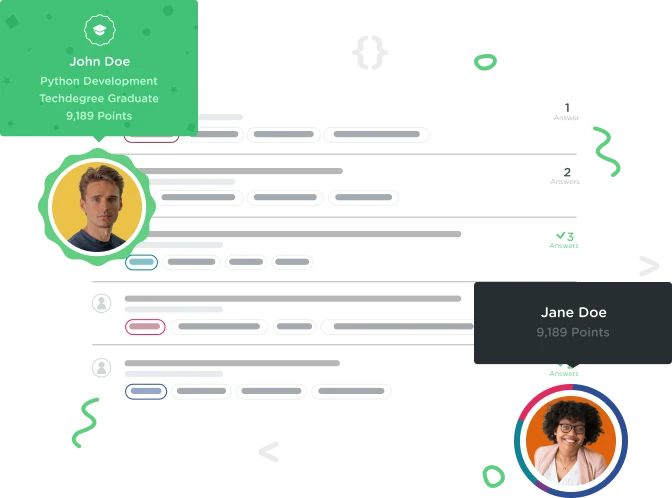

dexter foo
8,233 PointsDifference between var and let in creating an instance
Is there a difference in creating an instance as a variable or a constant? I understand it would not affect the stored properties of the instances but may i ask what is the difference between calling an instance a constant or a variable?
3 Answers

Ryan Jin
15,337 PointsOh, you are asking this. Great question! So I used to think of an instance of a class as objects like Integers, Doubles, etc. So Int is a type, Double is a type, NormalCars is also a type. So if you make a constant that has the type NormalCars, you cannot initialise it anymore, how ever, the properties of this type can be changeable if it is a variable. But you cannot reinitialise this constant. For example
class anInt {
var mNumber: Int?
init(number: Int) {
self.mNumber = number
}
}
let oneInteger = anInt(number: 45)
oneInteger.mNumber = 46 // You won't get an error, since the property mNumber is a variable
oneInteger = anInt(number: 100) // You will get an error, since oneInteger itself is a constant
Hope that helps :)

Ryan Jin
15,337 PointsA variable means that you can change it, it can be modified. In contrast, a constant cannot be modified, the value of permanent in the memory. Here is an example:
var mVariable: Int = 9 // Declaring a variable that equals nine
mVariable = 10 // You can modify the variable and change its value
mVariable = mVariable + 8 // You can modify it how ever you want as long as it stays as an int(Integer)
print(mVariable) // The console should print out 18, because mVariable = 10 changed its value to 10, and mVariable = mVariable + 8 adds 8 to itself, so 10 + 8 should get 18
let mConstant = 9
mConstant = 10 // You will get an error
print(mConstant) // If you delete the "mConstant = 10" above, you should get 9, which is its initial value
When you create an instance as a variable, it that this value of this property does not always stay the same. When you use a constant, it means that this property is a certain value no matter what you are going to do in the future. So for example, you might want to create a class called normalCar, the car might want to have an constant called numberOfWheels, because usually a car doesn't change the number of wheels, a normal car should have four wheels. But you might want to have a variable called yearsUsed, because the amount of time used will change.

dexter foo
8,233 PointsOh i get that if you create a variable, you can change its value but not for the case of a constant. But, what i want to know is that, say you've created a class, called NormalCars, with stored properties that are variables, var numberOfWheels: Double and var numberOfDoors: Double. Then you create an instance from that class. Does it make a difference if that instance is a variable or a constant? Meaning: Is there a difference between: Creating it as a variable: var Ford = NormalCars(numberOfWheels: 4, numberOfDoors: 4) and Creating it as a constant: let Ford = NormalCars(numberOfWheels: 4, numberOfDoors: 4)
Since you can just change the stored properties even if the instance is a constant.
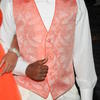
Rashii Henry
16,433 Points@dexter There actually is a difference in between creating that instance as a constant. When you create it as a constant you cannot modify the stored properties later on in your program. You'll only be able to use what it was assigned when you declared it. Obviously, its the opposite for declaring it as a var, you would be able to initialize it to (ex. numberOfDoors = 4). Then later on maybe in another AppState/GameState you will be allowed to change it using the dot notation. (ex: Ford.numberOfDoors = 6.).
I would probably suggest using var if you want to change the stored properties maybe at different times of your app. If you know beforehand that they will never change, then go ahead and declare them as constants and you'll have nothing to worry about.
dexter foo
8,233 Pointsdexter foo
8,233 PointsOH. Thanks! that helped a lot!