Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial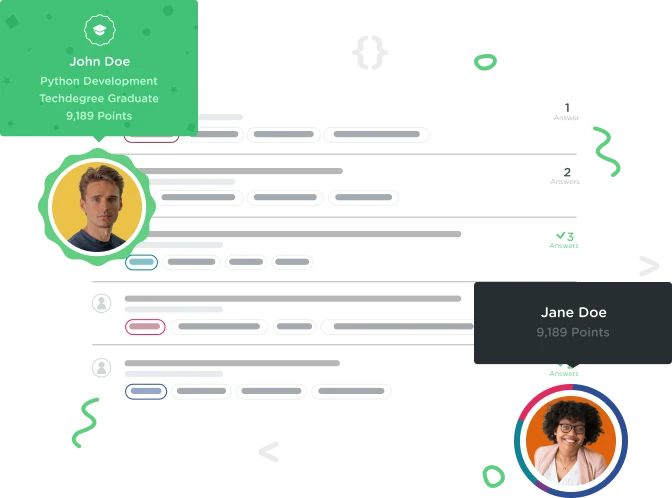
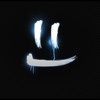
Grigorij Schleifer
10,365 PointsDifference betwen returning the list interface or List implementation.
Could someone explan me the diference? I don´t know what Craig is meaning ....
4 Answers

Craig Dennis
Treehouse TeacherHi Grigorji!
It's best practice to return the Interface so that at a later time you can change your implementation choice. This keeps things loosely coupled. When used as a parameter it makes a lot more sense, so let's try that:
public boolean compareTo(Comparable obj);
See there how the method is saying essentially, give me any type of object, as long as it can be compared(Comparable), I'll be happy." It doesn't matter what the object is.
By returning an interface you can change your mind on which implementation you use. With collections this is important because their design is based around choosing the implementation. As we have seen there are reasons you would choose one over the other. You want to be able to make that choice, without fear of breaking your code, or making large changes.
Remember, always imagine other people using your code. The less implementation details you expose, the more you'll be able to refactor under the hood.
That make sense?

Iain Diamond
29,379 PointsCraig, I think, is touching on the principle of Polymorphism. I'm not going to attempt to explain this in any depth (it's a fairly large, but interesting, topic), but what I will say is:
An interface is a contract. For example, the collection classes ArrayList, Vector, and LinkedList are all implementations that adhere to the List contract. That is, they agree to implement a specific set of methods, or in other words, behave in the same way.
This means if you have a method that uses an argument of an interface type then any object that meets the contract terms can be used in its place. For example, because an ArrayList implements the List interface, you can pass this into (or it return from, see example below) a method that takes a List argument.
List<String> getAllAuthors() {
ArrayList<String> authors = new ArrayList<>();
...
return authors;
}
One benefit of this is you can have an initial implementation of your method that uses an ArrayList, but change it later to use an alternative implementation, e.g. a LinkedList as both implementations behave the same way, without breaking the code (Craig's original point).
Hope this helps. iain

Craig Dennis
Treehouse TeacherOhhhh....the interface is the requirements, the contract, what must someone who claims to implement it must do. The implementation is the actual code that must have the methods defined in the interface.
That help?

Robert Morris
3,647 Pointsso basically the Interface holds methods that must be overridden in the Class if it is implementing that Interface in order to adhere to the contract?

Craig Dennis
Treehouse TeacherYes except the interface just contains the method signatures.