Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial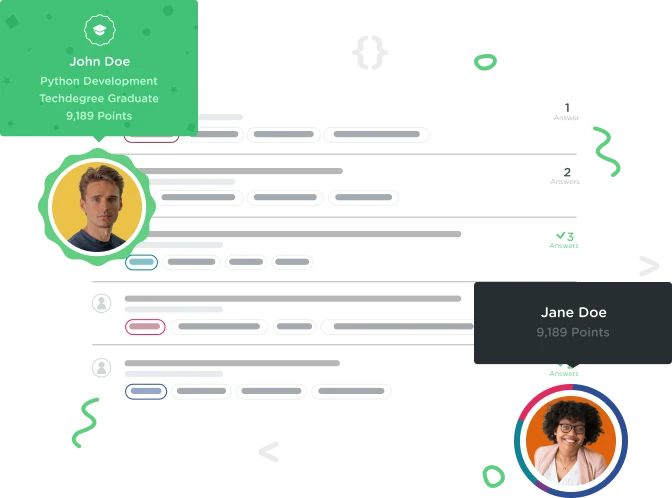
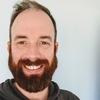
Jeremy Schaar
4,728 PointsDifference in using super() in Task 2 and Task 3 of challenge
Task 2/3 of the super() challenge is to override the add_item method and use super() to make sure the item still gets added to the list. That passes with this:
class Inventory:
def __init__(self):
self.slots = []
def add_item(self, item):
self.slots.append(item)
class SortedInventory(Inventory):
def add_item(self, item):
super().add_item(item)
self.slots.append(item)
Then task 3/3 asks us to sort the slot list. I thought this would work:
class SortedInventory(Inventory):
def add_item(self, item):
super().add_item(item)
self.slots.append(item)
self.slots.sort()
But I actually need to do this:
class SortedInventory(Inventory):
def add_item(self, item):
self.slots.append(item)
self.slots.sort()
I read in another place something about the super adding the item twice, but I'm pretty confused. If that's the case, why didn't the super() line cause a problem in the task 2/3? What changed in the third task?
Finally, both tasks 2 and 3 pass if I just do super() without the .add_item(), but Kenneth says in the video that "just using the word super isn't gonna do what we want it to do". Could someone elaborate on the difference between putting stuff after super() and just having it on its own?
Sorry! Long one! Many thanks in advance for any help!
1 Answer
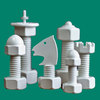
Steven Parker
230,274 PointsBoth using super to call the parent version of add_item, and performing the append yourself do the same job of adding the item. So from a functional standpoint you only need one or the other. But the challenge did specifically ask you to use super, so the first one would be the correct solution. Doing both does add the item twice, but apparently the check mechanism for task 2 isn't quite as stringent as it could be. But the test for task 3 caught it.
Calling "super()
" by itself returns a "proxy object" which isn't very useful except for accessing properties.
The difference between putting "super()" before a method name or using the method name itself is that with "super()" you're calling the method of the parent class, and with just the name you get the the one of the current class.
For example in this challenge, after you finish task 3, calling "add_item" in the SortedInventory class will add the item and sort the list, but calling "super().add_item" will just add the item to the end.
Jeremy Schaar
4,728 PointsJeremy Schaar
4,728 PointsFantastic explanation! Thanks so much.