Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial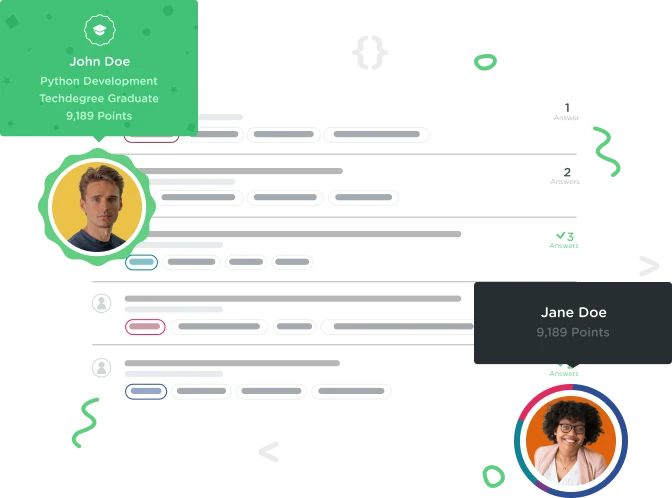
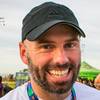
Brent Mayberry
1,029 PointsDifferences in way List<String> is created?
In the Sets video, Craig uses the List interface to create various List objects of type String. My question is: what difference is there between using the Array.asList implementation (do you call this an implementation? I'm trying to get the terms right.) versus the ArrayList implementation? Is the ArrayList implementation used when you want to copy an already existing List object?
List<String> frontEndLanguages = Arrays.asList("HTML", "CSS", "JavaScript");
and
List<String> allLanguages = new ArrayList<String>(frontEndLanguages); //is this extending a collection?
Why wouldn't the code below have worked?
List<String> allLanguages = new ArrayList<String>("HTML", "CSS", "JavaScript");
I know it throws a bunch of errors because I tried to do this in the REPL, but I'm having trouble grasping why. The error said "the constructor is not applicable" because the formal and actual arguments differ in length. But from what I can tell in the ArrayList documentation, (http://docs.oracle.com/javase/7/docs/api/java/util/ArrayList.html) the ArrayList constructors either take no arguments (to make a new, empty list) or they take an int (to set the list size) or they take a parameter "c" to extend a collection (which I have no clue as to what that means). Is extending the collection what happens when you pass the "frontEndLanguages" argument to create the "allLanguages" list? The documentation is confusing.
Thanks for your help.
2 Answers

miguelcastro2
Courses Plus Student 6,573 PointsI think it would help to understand what a Collection is and how it differs from an Array. A Collection in Java is an object that can store many objects. So if I had 3 String objects that each held a different name, I could store all of those string objects in a List and call this list studentNames. This List is a Collection which holds various String objects. Java has many kinds of Collections which you can use depending on the situation, List is one of the basic kinds of Collections you can use in Java.
You may be wondering how is a Collection different than an Array, since an Array can store items as well? An Array can only hold certain types of Java variables while a Collection can hold all kinds of Java objects. When working with Arrays, you must first declare the size of the Array before you can use it. If I declare an Array to have 10 elements, then 10 elements is all I can use. If I needed 11 I would have to declare that ahead of time. Sometimes we don't know how many elements we will need in advance. A Collection can grow and increase in size dynamically. We don't need to define it's size ahead of time and we can grow our Collection to fit our needs. That is just one of the examples on how they differ.
On to the code:
The Arrays.asList() method allows use convert an Array in to a List Collection. The Arrays.asList() will run first and create a collection of String objects, then assign that to our List collection.
List<String> frontEndLanguages = Arrays.asList("HTML", "CSS", "JavaScript");
Now that we have a List Collection, we can then pass that collection to the constructor of ArrayList()
List<String> allLanguages = new ArrayList<String>(frontEndLanguages);
ArrayList's constructor can receive a List Collection as an argument to set the allLanguages variable.
Lastly, you can do the same thing using this code, but it is not so readable and clean.
List<String> allLanguages = new ArrayList<String>(Arrays.asList("HTML", "CSS", "JavaScript"));
I strongly encourage you to read up on Collections because as a Java developer it will be a big part of the code you write.
Good luck!
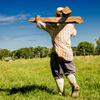
Nelson Fleig
23,674 PointsI still don't understand when to use the following methods for creating lists. Is there a preference or a difference? The output on java-repl seems to be the same.
List<String> list1 = new ArrayList<String>();
list1.add("foobar");
vs
List<String> list2 = Arrays.asList("foobar");
Is the Arrays.asList() method preferred for creating lists with a length greater than one?
Brent Mayberry
1,029 PointsBrent Mayberry
1,029 PointsMiguel! Thanks for explaining what Collections are. Your answer cleared everything up for me. I will start researching the documentation more so I can understand how these different implementations for interfaces work. Thanks again!