Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial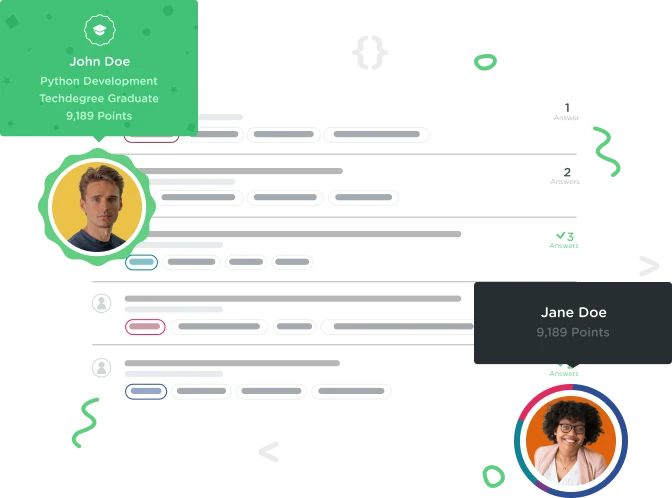

Johnny Lopez
4,643 Pointsdifferences of function declarations?
I'm not clear what the difference is between writing a function like: var addTask = function() {}, or function addTask(){}
why is it that we can assign one to addButton.onclick but not the other one?
3 Answers

Andrew Kiernan
26,892 PointsHey Johnny:
The difference between a function declaration(function addTask () {}
) and a function expression (var addTask = function () {}
) has to do with the way JavaScript code is executed.
A function declaration can be used before it is declared (this is through a process called function hoisting):
foo(); // "Hello, my name is Andrew"
function foo () {
console.log("Hello, my name is Andrew");
}
With a function expression, the expression must be declared before it is used, otherwise your program will throw an error:
foo(); // ReferenceError: foo is not defined
var foo = function () {
console.log("Hello, my name is Andrew");
};
Aside from that, the functions themselves work the same way.
Hope that helps!
-Andrew

John Hampton
8,200 PointsI understand the differences between the two types of function assignments and how they both use hoisting in different ways. What I'm not clear on is why the instructor used function expressions instead of function declarations with this course. As Johnny Lopez mentions above, at one point Andrew specifies that he will explain later why he chose to use function expressions. I don't recall it ever being elaborated on after that.
Can anyone else weigh in on this?
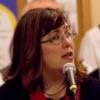
Tracy Trathen
10,468 PointsHe actually did explain it at 5:12 in the video:
"Now, if you remembered when I said that the way I was defining these, it looks maybe odd if you're just used to writing function and then the name. But I like to do this because I can do onclick, and type addTask, and I'm not tempted as much to do addTask with brackets. Now if you were creating a function like this, function addTask. You may be tempted to do this, and that will actually call the function there and then. You don't want it to do that, you want it to call when the user interacts and actually clicks on the button."

John Hampton
8,200 Points@Tracy Trathen Thanks for pointing that out, that clears things up a little.

Bradley Weston
Courses Plus Student 360 PointsYou can assign them both. They mean the same thing.
Most languages have anonymous function which is what these are.
var Cb = function () {};
el.onclick = Cb;
And your alternative way works too.
function Cb () {};
el.onclick = Cb;

Johnny Lopez
4,643 PointsOk, I guess I was confused because in the video he makes note that that is the reason why he wrote the functions like var Cb= function(){} and not in the other way, not completely sure what the instructor means there.