Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial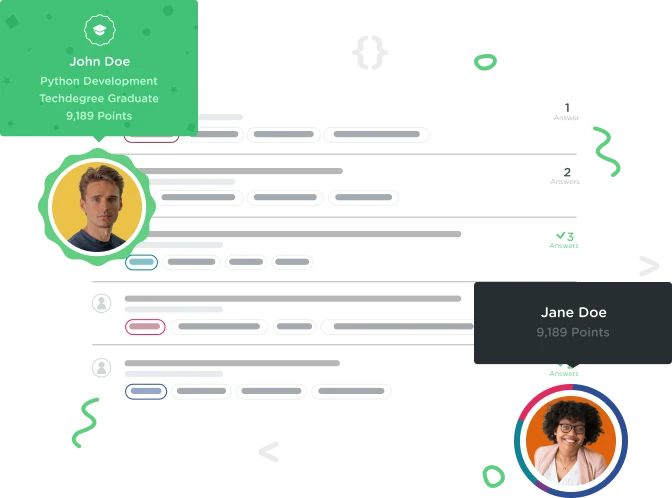
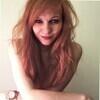
Silvia Ramos
Front End Web Development Techdegree Graduate 18,318 PointsDifferent approach
Hello! The teacher calculates the random number on the Math's operation. Can we create another variable that stores the subtraction between the higher number and the lower number instead?? and then process the Math's operation by only using that new variable? Thank you
2 Answers
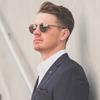
Cameron Sprague
11,272 PointsHi Silvia,
Yes, you can. Please see my code below as I create a newNumber variable to hold this value and use later in Math.floor().
// Collect input from a user
const inputLow = prompt('Please provide your lowest number');
const inputHigh = prompt('Please provide a number');
// Convert the input to a number
const lowNumber = parseInt(inputLow)
const highNumber = parseInt(inputHigh);
//Create a new variable to hold highNumber - lowNumber
const newNumber = highNumber - lowNumber
if (lowNumber && highNumber) {
// Use Math.random() and the user's number to generate a random number
const randomNumber = Math.floor( Math.random() * (newNumber + 1 ) ) + lowNumber;
// Create a message displaying the random number
console.log(`${randomNumber} is a random number between 1 and ${highNumber}.`);
} else {
console.log('You need to provide two numbers. Try again.');
}
You can even take lowNumber and move it up to the new variable. Please see the code below. Please let me know if you have any questions about the code below.
// Collect input from a user
const inputLow = prompt('Please provide your lowest number');
const inputHigh = prompt('Please provide a number');
// Convert the input to a number
const lowNumber = parseInt(inputLow)
const highNumber = parseInt(inputHigh);
//Create a new variable to hold highNumber - lowNumber
const newNumber = highNumber - lowNumber + lowNumber
if (lowNumber && highNumber) {
// Use Math.random() and the user's number to generate a random number
const randomNumber = Math.floor( Math.random() * (newNumber + 1 ) );
// Create a message displaying the random number
console.log(`${randomNumber} is a random number between 1 and ${highNumber}.`);
} else {
console.log('You need to provide two numbers. Try again.');
}
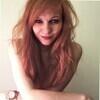
Silvia Ramos
Front End Web Development Techdegree Graduate 18,318 PointsHi Cameron,
Amazing stuff! thank you so much :)
To be honest, your code below does make more sense to me and it shows it much clearer. Thank you again for your help I am getting really slow at understanding JS, and I appreciate your prompt response and examples :)