Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial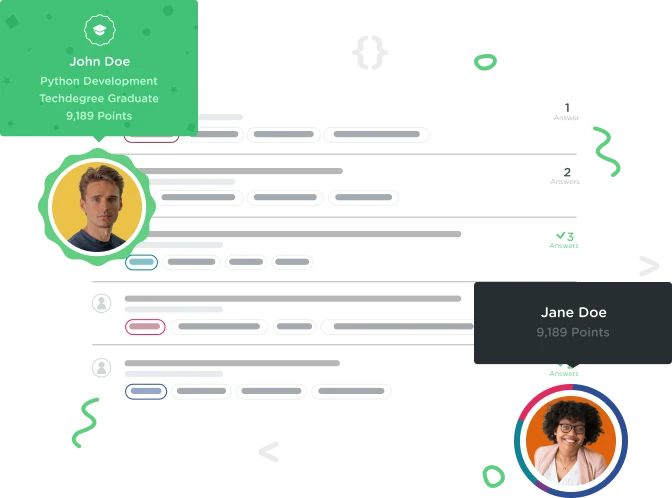
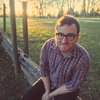
Christian Erickson
13,894 PointsDifferent approach/first attempt. It works, but I would love some feedback on if it is good coding practice, however.
What do you all think? I feel like it could be simplified a bit more in order to go along with the DRY principle, but not sure how.
var questions = [
['What is my first name?', 'christian'],
['What is my last name?', 'erickson'],
['What is my middle name?', 'stewart']
]
var score = 0;
for (var i = 0; i < 3; i += 1) {
var answer = prompt(questions[i][0])
answer = answer.toLowerCase();
if (answer == questions[i][1]){
score += 1
}
}
if (score === 1) {
document.write('You got 1 question right!');
} else {
document.write('You got ' + score + ' questions right!');
}
1 Answer
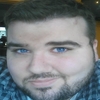
Marcus Parsons
15,719 PointsHey Christian,
You really want to try to make your code as dynamic and modular as possible when you're coding so that it can be used with other variations. We won't worry about being modular at this second, because I don't know what you do or don't know. But we should be worried with being dynamic. Right now, your for loop is very static because the conditional "i < 3" is static. If you add more questions to the array, you have to continuously update the for loop. That's not a good solution because it wastes your time. And time is precious, my friend.
So, instead of having an "i < 3" conditional, why don't we just use the array's length to determine when to stop? The array's length will always be one more than the maximum index of the array. You are seeing that now. You used 3 in there instead of 2 because 3 is one more than the last index in the array right now: 2.
It's really simple to do that. Just change "i < 3" to "i < questions.length":
var questions = [
['What is my first name?', 'Christian'],
['What is my last name?', 'Erickson'],
['What is my middle name?', 'Stewart']
]
var score = 0;
for (var i = 0; i < questions.length; i += 1) {
var answer = prompt(questions[i][0])
if (answer === questions[i][1]){
score += 1
}
}
if (score === 1) {
document.write('You got 1 question right!');
} else {
document.write('You got ' + score + ' questions right!');
}
Now you can put as many questions as you'd like within the array without ever having to calculate what the maximum number should be to loop through. This is dynamic coding!
Christian Erickson
13,894 PointsChristian Erickson
13,894 PointsYeah, totally forgot about that part. lol. Thanks man!
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsHaha no problem! We all need a reminder every once in a while. :)