Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial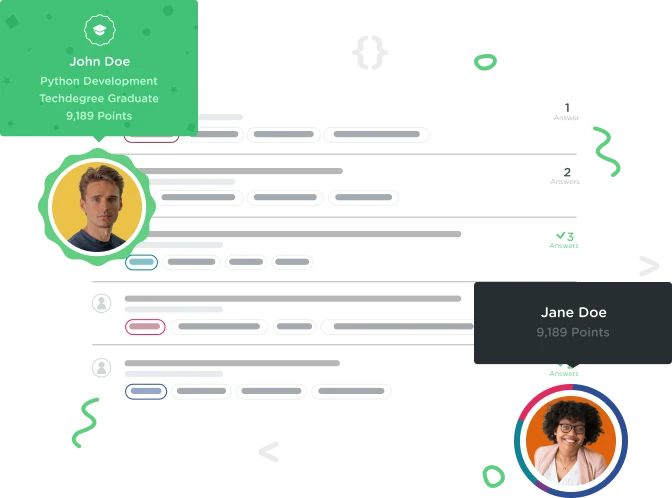

Michael Moore
7,121 PointsDifferent hangman code from theirs. Works. Has recursive function calls. Help improving?
Whenever they said hangman and I saw the requirements, I immediately paused the video and started writing my own. I am relatively experienced in Ruby but want to learn Python. I came up with this. The things I don't like about it is calling the same function from within the function, or passing all the variables through each function. I was trying to find a way around making them global variables. I did not want to make a bunch of globals, but rather, have as little global variables as possible. Any ways to improve this? And maybe explain why the coloring is all funky in my code? What I did wrong in pasting this code in here?
import random
guessed_letters = []
hangman_words = ["wonderful", "angry", "asfjaslkfjlaskfjlasfaa", "ilikepie", "feeeeeeeedme", "freeeeeeeeeeeeedoooooom", "itsfunctional", "butcouldbebetter", "dontcha", "luv", "dese", "werds"]
def main():
the_word = hangman_word()
rep = representation(the_word)
num = 6
the_rules(num)
print(rep)
the_noose(num, the_word, rep)
def game(num, the_word, rep):
thisround = False
try:
print(rep)
guess = str(input("Guess as if your life depends on it: ")).lower()
type_of_character = determining_correct_input(guess)
if type_of_character == False:
incorrect_input(num, the_word, rep)
if guess in guessed_letters:
print("Are you stoopid or sumthin?")
the_noose(num, the_word, rep)
else:
guessed_letters.append(guess)
i = 0
while i < len(the_word):
if the_word[i] == guess:
rep[i] = guess
thisround = True
i+=1
if rep == the_word:
game_won(the_word)
elif thisround == True:
game(num, the_word, rep)
else:
num -=1
the_noose(num, the_word, rep)
except:
print("You must want to hang")
the_noose(num, the_word, rep)
def the_rules(num):
print("""
You have {} chances before you are hung.
There are no numbers.
Guessing numbers will be counted against you, and you will be hung.
Here is your lifeline:""".format(num))
def representation(the_word):
rep = []
word_length = len(the_word)
while word_length > 0:
rep.append("X")
word_length -= 1
return rep
def game_won(the_word):
word = ''.join(the_word)
print("You got it, the word was {}.".format(word))
def hangman_word():
hangman_word = random.sample(hangman_words, 1)
hangman_word = hangman_word[0]
the_word = list(hangman_word)
return the_word
def the_noose(num, the_word, rep):
num -=1
if int(num) == 0:
game_over()
elif int(num)== 1:
miss_five(num, the_word, rep)
elif int(num)== 2:
miss_four(num, the_word, rep)
elif int(num)== 3:
miss_three(num, the_word, rep)
elif int(num)== 4:
miss_two(num, the_word, rep)
elif int(num)== 5:
miss_one(num, the_word, rep)
elif int(num)== 6:
miss_zero(num, the_word, rep)
def game_over():
print("""
_____
| |
O |
/|\ |
/ \ __|___
DIE DIE DIE DIE DIE""")
exit()
def miss_zero(num, the_word, rep):
print("""
_____
| |
|
|
__|___
""")
game(num, the_word, rep)
def miss_one(num, the_word, rep):
print("""
_____
| |
|
|
\ __|___
""")
game(num, the_word, rep)
def miss_two(num, the_word, rep):
print("""
_____
| |
|
|
/ \ __|___
""")
game(num, the_word, rep)
def miss_three(num, the_word, rep):
print("""
_____
| |
|
| |
/ \ __|___
""")
game(num, the_word, rep)
def miss_four(num, the_word, rep):
print("""
_____
| |
|
/| |
/ \ __|___
""")
game(num, the_word, rep)
def miss_five(num, the_word, rep):
print("""
_____
| |
|
/|\ |
/ \ __|___
""")
game(num, the_word, rep)
def determining_correct_input(guess):
try:
int(guess)
return False
except:
return True
def incorrect_input(num, the_word, rep):
print("You must want to hang")
num -=1
the_noose(num, the_word, rep)
main()