Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial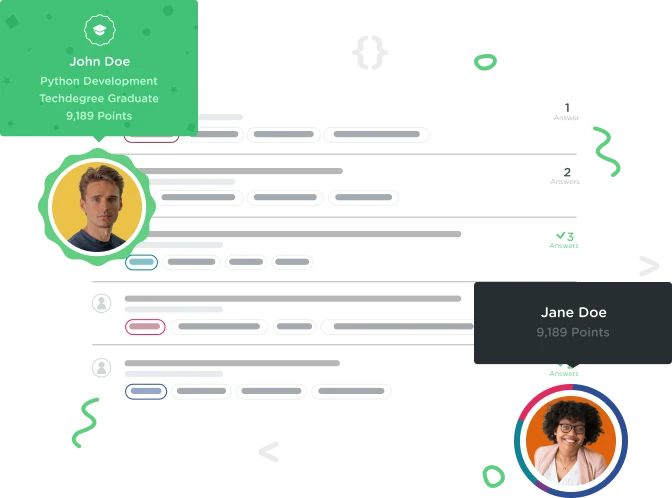

Michael Bailar
2,351 PointsDifferent so sharing.
Hi all,
This looked a bit different so I thought I'd share. I'd love any feedback you're willing to offer.
Thanks,
//declare variables and functions
var answers = [];
var score = 0;
var correct = [];
var incorrect = [];
var questions = [
["How many legs does an insect have?", 6],
["How many days are in a week?", 7],
["What is the meaning of life?", 42],
["What is 6 times 7?", 42]
]
//fun loop asking questions, keeping score, adding answers to new arrays, and formatting
for (i = 0; i < questions.length; i++){
answers.push('');
answers[i] = parseInt(prompt(questions[i][0]));
if(answers[i] === questions[i][1]) {
score +=1;
correct.push([i+1] + ". " + questions[i][0] + "<br>");
} else {
incorrect.push([i+1] + ". " + questions[i][0] + "<br>");
}
}
//Printing all messages. Arranged by line.
document.write("You got " + score + " question(s) right. <br>" +
"<h2>You got these questions right:</h2> <br>" +
correct.join("") + "<br>" +
"<h2>You got these questions wrong:</h2> <br>" +
incorrect.join("")
);
2 Answers
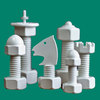
Steven Parker
231,275 PointsFor any non-trivial task, there will always be multiple ways to achieve the goal.
For the benefit of those who haven't just done this exercise themselves, how would you describe what's different?

Michael Bailar
2,351 PointsWell, I know four things I did differently.
I decided to put all my formatting into the actual JavaScript program so it could all be contained nice and neatly. (I did this prior to watching the second challenge video as I thought both parts were assigned in the first video.)
In my loop, I concatenated each answer element into a useable string that prints out a single answer with a line break tag This way, all I had to do was combine the elements in each array with a join command and they would would form a string that writes the correct and incorrect answers down in separate lines.
I declared, ahead of time that my variables we're arrays and told my program to create a new answer array slot for each question supplied.
I arranged the question printouts with the number related to the order the questions were asked rather than their order in each correct and incorrect answer array.
I think I would have done the formatting differently if I realized I ought to create html strings for the answer printouts.