Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial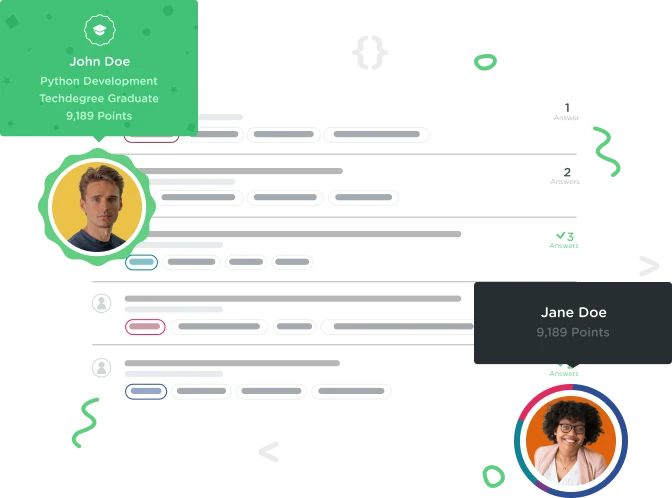

Derek Etnyre
20,822 PointsDifficult time thinking through the refactoring process
Having difficult time thinking through refactoring this line:
public Action<int, Func<int, int>> DisplayResult = delegate (int result, Func<int, int> function)
My first attempt was to put it like this:
public Func<int, Func<int, int>> DisplayResult = (int result, Func<int, int> function) =>
but keep thinking the two Func<int, int> 's can be simplified to something like this:
public Func<int, int> DisplayResult = (int result, int function) =>
Neither one is right - think my first attempt is closer to the solution.
Any suggestions on how to think through the refactoring process on this one?
using System;
namespace Treehouse.CodeChallenges
{
public class Program
{
public Func<int, int> Square = (int number) =>
{
return number * number;
};
public Func<int, int> DisplayResult = (int result, int function) =>
{
Console.WriteLine(function(result));
};
static void Main(string[] args)
{
}
}
}
2 Answers
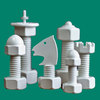
Steven Parker
229,744 Points
You almost got it on the 2nd attempt.
You just forgot that argument types for lambda's are inferred, not specified. Also, don't change the type of DisplayResult:
public Action<int, Func<int, int>> DisplayResult = (result, function) =>
Oddly, it apparently let you get away with it on task 1.
Actually, you can compact things even more by eliminating the blocks (and the return on task 1:
public Func<int, int> Square = number => number * number;
public Action<int, Func<int, int>> DisplayResult = (result, function) =>
Console.WriteLine(function(result));

Derek Etnyre
20,822 PointsJust figured it out - should have left the Func <int, int> in place - like this:
public Action<int, Func<int, int>> DisplayResult = (result, function) =>
{
Console.WriteLine(function(result));
};
Derek Etnyre
20,822 PointsDerek Etnyre
20,822 PointsThanks. Still something off that I'm not getting.
Have code looking like this now:
but still not able to compile.
Put code into Visual Studio and it tells me: 'Delegate 'Func <int, int>' does not take 2 arguments.
Tells me I'm not fully understaning the Lambdas....