Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial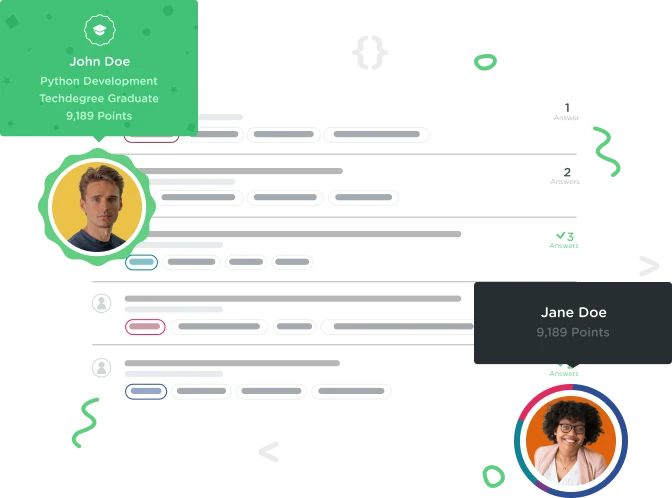

yashita mittal
4,678 PointsDifficulty in printing array of objects to screen
I have 2 files - student.js and index.html
student.js
var students = [
{ name: "Sonam",
track: "Front-End development",
achievements: 10,
points: 800 },
{ name: "Neha",
track: "ios",
achievements: 10,
points: 800 },
{ name: "Amit",
track: "Mechanical",
achievements: 10,
points: 800 },
{ name: "Aarav",
track: "Toddler",
achievements: 10,
points: 800 },
{ name: "Saurabh",
track: "Front-End development",
achievements: 10,
points: 800 },
];
//print the students details
function printRecord(html) {
var ref = document.getElementById('output');
ref.innerHTML = html;
}
//Run a for loop to get all the information about a student
var html;
for(var i = 0; i < students.length; i++) {
html = "<h2> Student " + students[i].name + "</h2>";
html += "<p>Track " + students[i].track + "</p>";
html += "<p>Achievements " + students[i].achievements + "</p>";
html += "<p>Points " + students[i].points + "</p>";
//Print
printRecord(html);
}
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Students</title>
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<h1>Students</h1>
<div id="output">
</div>
<script src="js/students.js"></script>
</body>
</html>
When I run this on browser, only the last object of the array is printed. I don't understand the reason for this behaviour. If I change the code in printRecord() function : ref.innerHTML = html; to console.log(html) then all the records from the array get printed on the console Can someone please explain me what am I doing wrong.

Kathryn Notson
25,941 Pointsyashita mittal:
I wouldn't use "var html;" as an empty variable. You might be confusing the browser. Use another name for the variable. See if that helps.
The other problem I see is using "Print printRecord(html)." It should be formatted like this "print(variable);". Whatever variable name you substitute for "html", use the same name in the "print()" function.
Hope that helps.
3 Answers
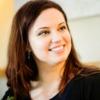
Emily Carey
7,954 PointsJesus was on the right lines.. you're overwriting the html you're putting out with the very last iteration of the loop.
To fix this, you need to change...
ref.innerHTML = html;
to...
ref.innerHTML += html;
This means that everytime you send a loop iteration to the function to print the student's data out, it's adding to the html not overwriting what was previously there. You could use child nodes also to achieve this but for simplicity, this should be your solution :)
Else, by placing the printRecord(html); outside of your loop should also fix this BUT you need to replace the + on the first h2 to += as other people's comments.

Meghana Rawate
7,144 PointsChange this html = "<h2> Student " + students[i].name + "</h2>";
to html += "<h2> Student " + students[i].name + "</h2>";
You are creating a concatenated string as you add to the html variable. Plus you are iterating it through a loop. So in each iteration it reaches the line without the += and overwrites the content. That is why you end up seeing only the last object that has been iterated.
So it is like: Sonam //assign
- Front-End-Develpment // concatenate
- 10 // concatenate
- 800 // concatenate
then assign! ..so the record above is wiped out and this continues
Neha // assign
- ios //concatenate
- 10 //concatenate
- 800 //concatenate
Then again assign! ... so the above record is wiped out
This goes on till the last record and that is what you end up seeing.
I hope my silly explanation helps :) Happy coding!

Kathryn Notson
25,941 Pointsyashita mittal:
They are all correct. One thing to remember is to look at your syntax carefully (punctuation marks & mathematical operators). It's critical they are correct as they affect your code. (I've made 6 very humorous syntax errors which did some very odd things. If I could have made a video of them, everyone would laugh!) It would be good to write down syntax errors for future reference. It helps to be very detailed oriented, a good typist/keyboardist, & editor, too.
Happy coding!
Jesus Mendoza
23,288 PointsJesus Mendoza
23,288 PointsYour code is missing a "+" here:
html += "<h2> Student " + students[i].name + "</h2>";
And because of that, every time your loop runs then it deletes everything from the previous loop, it's not concatenating anything but setting a new value.