Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial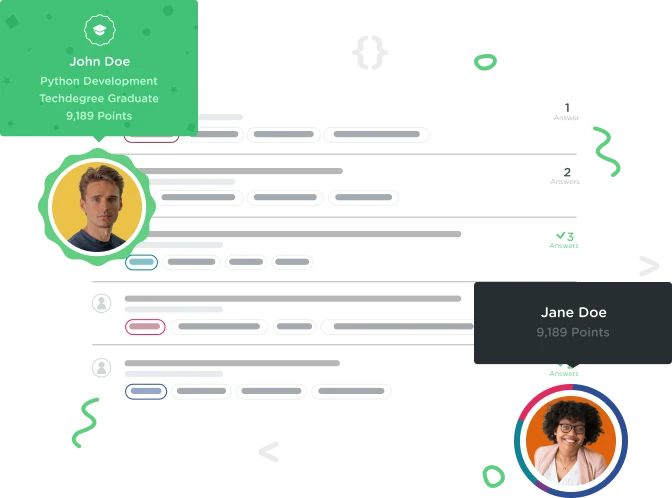
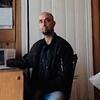
Louis Sankey
22,595 PointsDifficulty making an API call
So I'm trying to make my first API call for a new app I'm trying to develop. The problem is that I don't understand exactly what format the url and api key are supposed to be in. In the video the key is part of the url. Therefor I have tried:
String apiKey = "iSXHqcobFZlEJaWvs5mnk9eQU0fOpKMP"; String proBasketballApiUrl = "https://probasketballapi.com/" + apiKey;
if(isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(proBasketballApiUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
}
@Override
public void onResponse(Response response) throws IOException {
try {
Log.v(TAG, response.body().string());
if (response.isSuccessful()) {
} else {
alertUserAboutError();
}
} catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
});
}
else{
Toast.makeText(this, "Network is unavailable!", Toast.LENGTH_LONG).show();
}
But this is not returning json. The documentation on the website with the data I am trying to access says this:
"Authentication To make calls to the ProBasketball API, you must make a POST request to the specified URL. In this request you must pass along your API key, and that is it. You now have access to the API!
Of course you can pass along arguments other than just your API key, in fact, it is encouraged that you do so. It will help narrow down data that will be returned.
All responses will be in the JSON format."
Then they give this example in php, which I am unable to interpret exactly.
<?php
$url = 'https://probasketballapi.com/teams';
$api_key = 'YOURAPI_KEY_';
$query_string = 'api_key=' . $api_key . '&team_abbrv=BOS';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_POSTFIELDS, $query_string); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
$result = curl_exec($ch);
curl_close($ch);
echo $result;
?>
I'm hoping a pro can shed some light on this for me. Thanks
3 Answers

Kane Stoboi
21,919 PointsI think I've found your problem.
There are a couple of ways to provide parameters to a web server. One is by using the GET method which is what I believe is covered in most of the treehouse courses. I think it might also the most common method. Another way to provide parameters is using the POST method. This is usually used when submitting web forms. It seems like the api at probasketballapi.com uses the POST method for you to add the parameters and then "POST" them to the server rather than just having them in a url.
Here's a link to some okHttp examples of different ways of requesting data https://github.com/square/okhttp/wiki/Recipes.
Here is a method to submit the parameters to probasketballapi.com
public void run() throws Exception {
String api_key = "iSXHqcobFZlEJaWvs5mnk9eQU0fOpKMP";
//Here is where you will need to edit parameters for the api, just like filling out a form on a website
RequestBody formBody = new FormEncodingBuilder()
.add("api_key", api_key)
.build();
//Builds the request
final Request request = new Request.Builder()
.url("https://probasketballapi.com/teams/")
.post(formBody)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
}
@Override
public void onResponse(Response response) throws IOException {
Log.v(TAG, response.body().string());
}
});
You should also add this variable and constant to the class
public static final MediaType MEDIA_TYPE_MARKDOWN = MediaType.parse("text/x-markdown; charset=utf-8");
private final OkHttpClient client = new OkHttpClient();
I hope this helps.
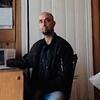
Louis Sankey
22,595 PointsThank you! I can see on my account page that when I run this code it is making the api call successfully. The only problem I am still having is that when I Log response.body() I am still not seeing the code in the logcat. I am wondering, when you ran this code yourself were you able to see the json data?
Thanks again for the help!

Kane Stoboi
21,919 PointsNo problem Louis. I was able to log the JSON data to the logical when I ran it. Sometimes when there is a large amount displayed in a log it can be minimised. Have you tried searching for the tag?
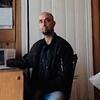
Louis Sankey
22,595 PointsAfter searching for the json data in logcat and scratching my head for the last day and a half I finally ran the code in a new project and it worked perfectly. In my original project I have some static json loading from my assets folder and I think it may be conflicting somehow with the api call. Anyway, I have enough now to continue working on my project. Thank you again, Kane, I would still be stuck if not for the help.