Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial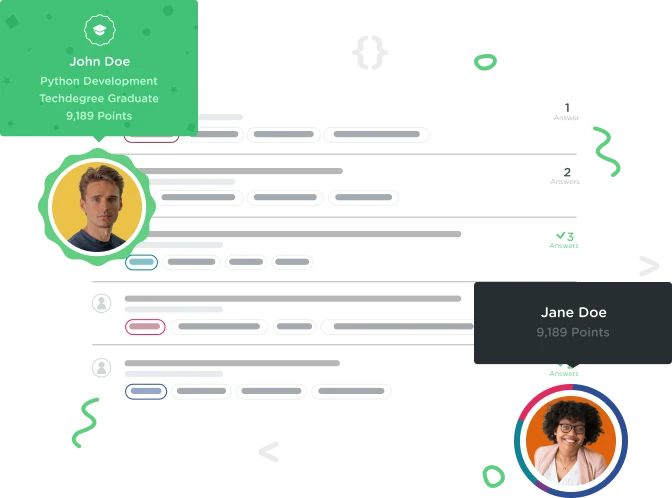

Laknath Gunathilake
1,860 Pointsdifficulty passing stat challenge
when I run the following code
def stats(my_dict):
stat=[]
for teacher in my_dict:
count=len(my_dict[teacher])
stat.append(teacher+str(count))
I get something like
['Goerge1] where as what I need is ['Goerge','1']
```teachers.py
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(my_dict):
max_teacher=''
max_count=0
for teacher in my_dict:
count=len(my_dict[teacher])
if count>max_count:
max_teacher=teacher
max_count=count
return max_teacher
def num_teachers(my_dict):
return len(my_dict.keys())
def stats(my_dict):
stat=[]
for teacher in my_dict:
count=len(my_dict[teacher])
stat.append(teacher+str(count))
2 Answers
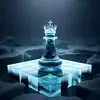
crosscheckking
19,866 PointsIt wants you to return a list of lists. So you will need to ".append([teacher, count])". This will append the list of the teacher name and class count to the list. Also it wants you to keep the count as an int rather than a string. Also, don't forget about the return statement(:
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(my_dict):
max_teacher=''
max_count=0
for teacher in my_dict:
count=len(my_dict[teacher])
if count>max_count:
max_teacher=teacher
max_count=count
return max_teacher
def num_teachers(my_dict):
return len(my_dict.keys())
def stats(my_dict):
stat=[]
for teacher in my_dict.keys():
count=len(my_dict[teacher])
stat.append([teacher, count])
return stat

Wairton Rebouças
8,225 Points"Now, create a function named stats that takes the teacher dictionary. Return a list of lists in the format [<teacher name>, <number of classes>]. For example, one item in the list would be ['Dave McFarland', 1]."
you got ['Goerge1'] instead of ['Goerge','1'] because you're doing stat.append(teacher+str(count)), adding just one string with the two items concatenated instead of adding a list with two elements [teacher, count]. Note that you don't need to convert count to string and also remember of returning the 'stat' variable at the end of your function.