Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial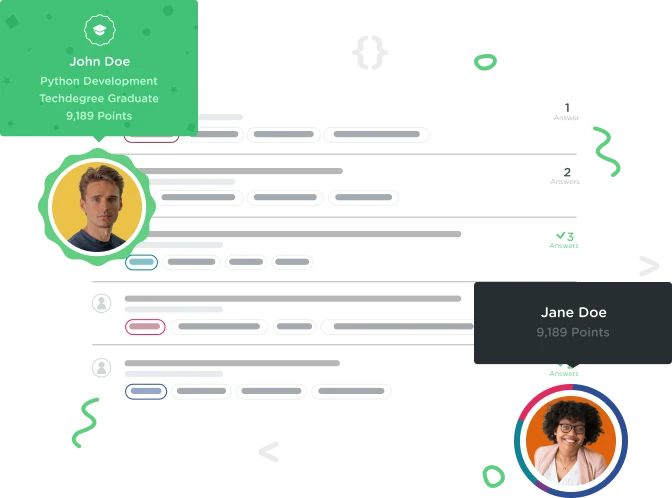
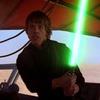
darrian thomas
8,481 PointsDifficulty: Timestamp Ordering Challenge
Not sure what I should do I think I'm lost in Python Dates and Times I don't even know how to start this challenge lol
I'm wondering if I should try a different language and come back to this. What language do you think is like Python that will help me understand more of what is going on
I think I should be trying to practice some of these things outside of treehouse maybe that would help cement some knowledge maybe I am forgetting things
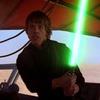
darrian thomas
8,481 Pointsthanks for the quick response. i actually really like python but find myself getting stuck and having to find help on the forums often but the most problems ive had have been in this time course. im not a big fan of messing with times. for the challenge im not even sure where to start.
"Create a function named timestamp_oldest that takes any number of POSIX timestamp arguments. Return the oldest one as a datetime object. Remember, POSIX timestamps are floats and lists have a .sort() method."
ok so I need to make a function that takes 'any number of posix timestamp arguments' from there on im pretty much lost
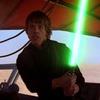
darrian thomas
8,481 Pointsok so i found your answer to the problem on another post about this challenge, @Chris Freeman
import datetime
def timestamp_oldest(*args):
oldest_time = list(args)
oldest_time.sort()
return datetime.datetime.timestamp(oldest_time[0])
i think i understand what you did but can you walk me through step by step whats going on? Here's what I think is happening.. 1) unpacking tuples from args into function 2) setting the tuples into a list stored in a variable 3) sorting that variable from least to greatest in value 4) finally returning the oldest value [0] index from the sorted list in a timestamp
the thing is I had to look up the answer and even though I know how it works leaves me frustrated that I could not think of it myself. have any tips on things like this? I hope this is a common problem for most beginners and that soon I'll get over it

Gavin Ralston
28,770 PointsThe way you start to learn to figure these things out on your own is by working on them. Some bits more than others. :)
Everybody googles or uses stack overflow or consults the docs. You'll never get away with not doing that kind of research, but you'll find some things come up again and again, and you'll start to just develop an intuition.
Plus you'll be more mindful of things like "I'm getting this type of object back, so I'll do this, this, then this to get what I want" rather than having to be so deliberate about the process.
1 Answer
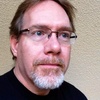
Chris Freeman
Treehouse Moderator 68,423 Pointsi think i understand what you did but can you walk me through step by step whats going on?
Sure.
Here's what I think is happening..
1) unpacking tuples from args into function
Think of *args
as a receiver of an arbitrary number of arguments.
2) setting the tuples into a list stored in a variable
Yes. Calling list(args)
sets all the arguments into a list.
3) sorting that variable from least to greatest in value
Yes. smallest value to largest value is the default sort.
4) finally returning the oldest value [0] index from the sorted list in a timestamp
Correct. You've got it.
import datetime
def timestamp_oldest(*args):
oldest_time = list(args)
oldest_time.sort()
return datetime.datetime.fromtimestamp(oldest_time[0])
Edit fixed typo. Should be .fromtimestamp()
not .timestamp()
the thing is I had to look up the answer and even though I know how it works leaves me frustrated that I could not think of it myself. have any tips on things like this? I hope this is a common problem for most beginners and that soon I'll get over it.
It is a beginner's issue. Not to worry. As you are exposed to more code styles and packages (like datetime, pytz, random, math, etc) you start to see a flow.
Like word morphing puzzles, many times you know what goes in and what comes out.. What you're left with is figuring out the steps in between. A "how do I get there from here" game.

anhedonicblonde
3,133 PointsWhen I use the solution above I get an error: "descriptor timestamp requires a datetime.datetime object but received a float." I was completely unsure how to go about this one, as well ... now I'm really thrown.
What am I missing? thanks
Nevermind - I got it. The last line is missing "from" in timestamp. It should read
return datetime.datetime.fromtimestamp(oldest_time)
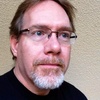
Chris Freeman
Treehouse Moderator 68,423 PointsThanks! I have updated the Answer to fix the typo.

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsThanks for your help, Chris!
If you wouldn't mind, can you explain to me why the function below would not work?
def timestamp_oldest(*args):
sorted_list = args.sort()
return datetime.datetime.fromtimestamp(sorted_list[0])
When I do this I get this error:
'tuple' object has no attribute 'sort'
Several websites like this one say that *args passes a list. So, why do I then have to cast *args to a list like in your solution above? Shouldn't args be able to use the .sort() method if it's already a list like these websites say?
Thanks!
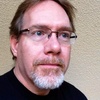
Chris Freeman
Treehouse Moderator 68,423 PointsChris Jones, the website you reference is using "list" in a colloquial fashion and not the literal Python list
object sense.
Argument lists are indeed tuples:
>>> def argpass(*args):
... print(type(args))
...
>>> argpass(1, 2, 3)
<class 'tuple'>
Since tuples are immutable, the sort()
method is not available.
Also, be aware that list.sort()
sorts a list "in place" modifying the original list and does not return a value. If you had first converted args
to a list before trying to sort, sorted_list
would have been assigned None
. This would raise an error in the return statement because None
can't be subscripted as sorted_list[0]
Braking it down, this would work:
def timestamp_oldest(*args):
listargs = list(args)
listargs.sort()
return datetime.datetime.fromtimestamp(listargs[0])

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsAh, that makes sense. Thanks for your explanation:)! It's much appreciated!
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsTimestamps are just one small aspect of Python. It's better to figure out what part is difficult then ask in the forum.
Can you add information about what terms, or code, or concepts are not clear?
We're all happy to help!