Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial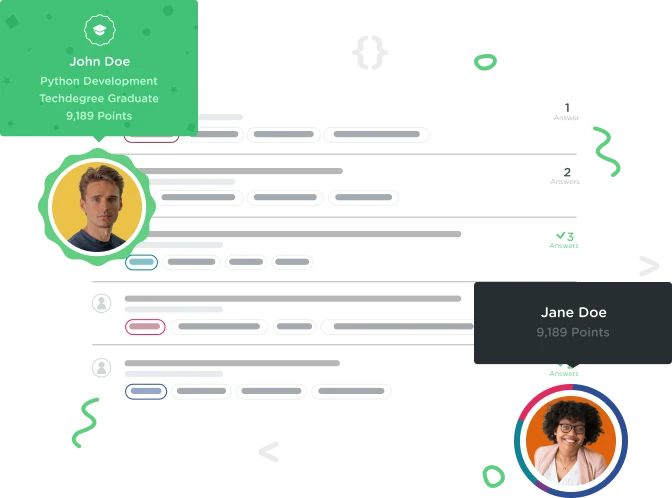

Ryan Malkmus
2,053 Pointsdifficulty understanding kwargs
hey guys, i'm having a hard time understanding kwargs. i feel like I can grasp everything in this video up to the point that kwargs get introduced. Is there a resource that someone can turn me to that may allow me to understand how kwargs and get works a little bit better? I'm feeling discouraged :(
4 Answers
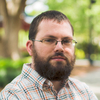
Kenneth Love
Treehouse Guest Teacher.iteritems()
is actually a Python 2 feature, not Python 3. In 3 we just use .items()
and it's still a generator (don't worry about that word for now).
Kwargs works for both unpacking that dictionary, like in Maciej Sitko 's example and another way that's often a bit more useful.
def person(**kwargs):
output = []
for key, value in kwargs.items():
output.append("This person's {} is {}".format(key, value))
return output
So now I can call person
with whatever keyword arguments I want, just like I had defined a bunch of arguments.
>>> person(name="Kenneth", job="Teacher", company="Treehouse")
["This person's name is Kenneth", "This person's job is Teacher", "This person's company is Treehouse"]
Being able to take a dictionary is awesome. Being able to take any number of keyword arguments and use them as a dictionary is often more awesome.

Maciej Sitko
16,164 PointsKwargs are just a fancy way of saying that it is about using multiple keyword arguments in form of array, hence two asterisks used. You could, of course, get away without using the word 'kwargs', but it is a convention used among Python users. It is enough to use asterisk and any word you like, but its highly advisable to stick with Python convention.Python is all about strict, and specific conventions.
In general, args are arrays that consist of standalone items, while kwargs are dictionaries with key and value.
Look at the function, the difference is show in comparison of args to kwargs:
1) Args
def args_me(*args):
for arg in args:
print "And here goes argument: ", arg, "\n"
args_me('mum', 'dad', 'brother', 'sister')
This will output:
And here goes argument: mum
And here goes argument: dad
And here goes argument: brother
And here goes argument: sister
2) Kwargs on the other hand:
kwargs = {mother : "mum", sister : "sis", father : "dad", brother : "bro"}
def kwargs_me(**kwargs):
for key, value in kwargs.iteritems():
print "And here goes kwargument: ", str(key), " = ", value, "\n"
kwarg_me(kwargs)
And this will output:
And here goes kwargument: mother = mum
And here goes kwargument: sister = sis
And here goes kwargument: father = dad
And here goes kwargument: brother = bro
Hope that I helped a bit.

Ryan Malkmus
2,053 Pointsthank you, it does help a little. I can't get any further from understanding, is how I like to try and think about it.
So, please don't get too frustrated with me but, what does iteritems() mean? Could you have used kwargs.items() to call the value of the dictionary? Also, what is the /n doing?
I think it's the dictionary concept that I need to work on maybe. I think that arrays are easy to see what's happening. But dictionary's get confusing for me right now when I'm trying to think of how to call the certain keys and values.

Maciej Sitko
16,164 Pointsiteritems() return the iterator over the items in the list, step by step, pair by pair, whereas items() return the whole copy of dictionary's keys and values as a full-blown list the moment when you call it.
I guess you can imagine that items() is more memory consuming especially when you deal with big dictionaries, because the program builds them at once. It depends what you want to do, items() could be handy, for example, if you were to check the overall list's length.

Ryan Malkmus
2,053 PointsOh, okay, cool. I see, thank you
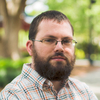
Kenneth Love
Treehouse Guest TeacherNope, dict.get()
is handy inside and outside of classes. You're right about it letting us have a default value if the key isn't found (or, if you just want None
, that's the default value returned). It doesn't let us set a default value, though.
Maciej Sitko
16,164 PointsMaciej Sitko
16,164 PointsOh yes, that's right I totally forgot/ignored the fact about using multiple keyword arguments in my response.
Ryan Malkmus
2,053 PointsRyan Malkmus
2,053 PointsWhat about using "get()". is that something that normally only gets used inside of a class? I think that get(), gets the key and value of a dictionary. It's useful because, you must set defaults for it. That way, if you don't want to specify a different value for any given key, you will get your keys and values assigned to a new instance of your class without getting any errors. Does that sound like I understand get()? I feel like i'm on the edge of understanding a lot of these python concepts. Its frustrating because I'm a slow learner. It's easy for me to look at code and get it what's happening when you guys write it, but it's way different to try and produce it out of thin air and have it be as clean and functional as you guys can make it.
Graham Mackenzie
2,747 PointsGraham Mackenzie
2,747 PointsSo, basically, what's happening here is that whenever a user makes an instance of a class, any keyword variables they set in the instantiation are sent to the class call as a dictionary, and putting
**kwargs
in the class's__init__
method allows that dictionary to be unpacked as a collection of keywords and values which then override any default attributes, am I understanding this right?Thanks! Graham