Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial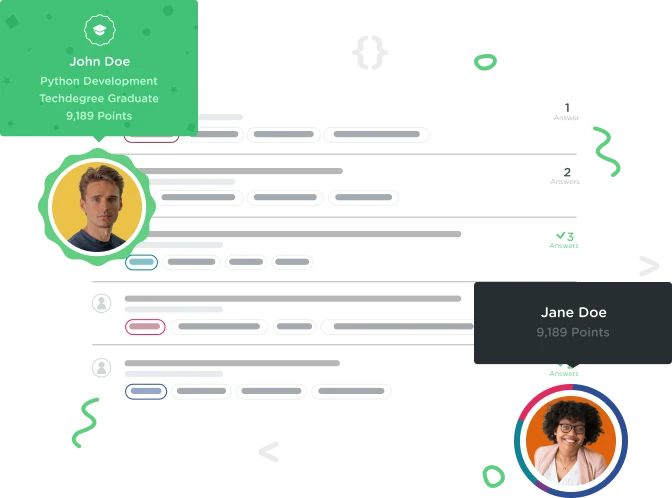
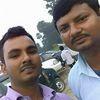
shubhamkt
11,675 Pointsdisemvowel
I am trying to remove the vowels one by one but it is not working. Any suggestions
1 Answer
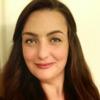
Jennifer Nordell
Treehouse TeacherHi there! Unfortunately, this approach will not work as it doesn't take into account words with multiple instances of the same vowel. For example, the word "balloon". If I were to send that in, it would only remove one instance of "o" and what would be returned would be "bllon", which still contains one vowel. So I'm going to give some pseudocode here that hopefully will help you understand the the approach I used.
create a list that contains all vowels that need to *not* be in the returned string
create a new empty string
loop through the word
if the letter is not in the vowels list
append it to the new empty string
return the new string with all letters that are not in the vowels list
Hope this helps!
shubhamkt
11,675 Pointsshubhamkt
11,675 PointsModerator edited: Added markdown so the code renders properly in the forums.
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse Teachershubhamkumartrivedi There are a couple of ways to do this. In the code you posted you are turning word into a list and then appending the letter onto an empty array. The result will be that
string
will contain an array of letters instead of a string of characters. In this case you will need to use this line before you returnstring
.string = ''.join(string)
Alternatively, you could skip that all together and do something slightly shorter. This was my approach:
In the second approach there is no need to turn the word into a list nor join the elements of a list to form a string. Rather we start with a string and keep it that way through the duration.
Hope this helps!