Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial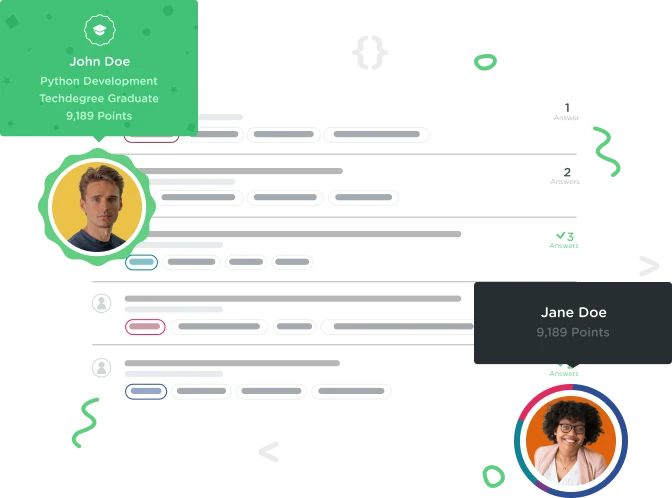

Andrew Bickham
1,461 Pointsdisemvowel
could someone possibly check my coding to see where I could make some improvements
def disemvowel(word):
for letter in word:
if letter.lower() in "aeiou":
if letter.upper():
letter.remove("aeiou")
return word
5 Answers
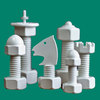
Steven Parker
231,545 PointsAs you probably know already from the "Bummer" message, a string has no remove method. Also, even if you convert the word into a list, the "remove" function can only be used with one letter at a time.
And while many folks do solve this by converting to and from a list, you dont have to do that. There's a string function called "replace" that could be handy if you wanted to solve it without conversion.
Two more hints, be sure to return only after you loop is finished, and return the modified word and not the original.
Give it another shot, and write again if you need more help.

Andrew Bickham
1,461 Pointsdef disemvowel(word): for letter in word: if letter.lower() in "aeiou": if letter.upper(): letter.replace("aeiou","") return letter
so steven I have my for loop that loops through each "letter" one at a time correct? yet obviously something isn't right or is missing so if you could just take another look at and see what could be the problem. thank you
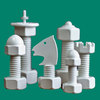
Steven Parker
231,545 PointsSee the comment I added to my answer. And if you need to post code again, please use "Markdown" formatting. There's a "cheat sheet" below, and you can also watch this video on code formatting.

Andrew Bickham
1,461 Points'''python def disemvowel(word): for letter in word: if letter.lower() in "aeiou": letter.replace("a" "e" "i" "o" "u", "") return letter
''' hey steven we go be real good friends when this is all said and done but i made some few changes and when i got to see if i cleared the code, basically the only letter that remains is the first letter and all the other letters are removed. i still cant completely see what im missing
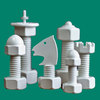
Steven Parker
231,545 PointsThose 3 marks that indicate code should be accents (`), not apostrophes (').

Andrew Bickham
1,461 Pointsdef disemvowel(word):
for letter in word:
if letter.lower() in "aeiou":
letter.replace("a" "e" "i" "o" "u", "")
return letter
forgive me
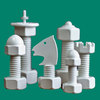
Steven Parker
231,545 PointsYay! Now we can actually see it. And I notice that the "return" is indented to place it inside the loop, which will stop the function too soon. And remember my previous hint: ""replace", like "remove", will only work for one letter at a time".
And think about what the loop is doing. If you pick each letter out of the word, is it the individual letter you want to perform "replace" on? And do you only want to return one letter?
Plus, this is a very popular topic that people ask questions about. You might want to look at some of those other questions and their answers.

Andrew Bickham
1,461 Pointsdef disemvowel(word):
vowels = "aeiou"
for letter in word:
if letter.lower() == vowels:
if letter.lower == "a":
letter.replace("a","")
if letter.lower == "e":
letter.replace("e","")
if letter.lower == "i":
letter.replace("i","")
if letter.lower == "o":
letter.replace("o","")
if letter.lower == "u":
letter.replace("u","")
return letter
so i made some adjustments, it stills keep giving me just that last letter everytime i try and clear the challenge but i am working on a whole new code to complete this challenge but i dont want to just give up on this one but i am ready for this challenge to be cleared
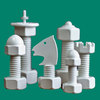
Steven Parker
231,545 PointsThat's why I asked last time " And do you only want to return one letter?". The return statement is currently "return letter
", but "letter" only represents one letter in the loop.
So I was suggesting that you might want to return the whole word. And do the removing on it also instead of the letter.
Andrew Bickham
1,461 PointsAndrew Bickham
1,461 Pointshey steven, so I made some modifications and what keeps tripping me up is the replace function, in my code I wrote as such letter.replace("aeiou",""); i put in a empty string because i wasn't sure what to replace the vowels with. when i go to see if i cleared the challenge the message it gives me is that instead of removing the only the vowels it removes all the letters except for the last one, you said a lot of people clear this challenge by converting it to a list, well being different is what wakes me up every morning, so i would prefer to clear the challenge with the replace function
Steven Parker
231,545 PointsSteven Parker
231,545 PointsThe "replace" function would be my choice also, but you will probably want to create a loop to make it take out one letter at a time. Give that a try, and if you still have trouble post your complete code back here so we can take another look.
Steven Parker
231,545 PointsSteven Parker
231,545 PointsYou seem to be headed in the right direction, here's a few more hints:
if letter.upper()
" isn't complete, did you mean to compare it to something?