Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial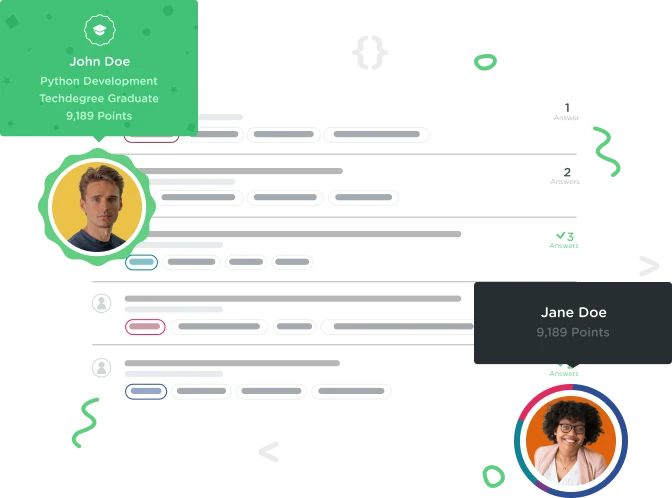

Kyla Hill
992 Pointsdisemvowel
I have tried everything I can think of, and now I've over thought so much I don't even know where to start. Please help.
def disemvowel(word):
return word
1 Answer
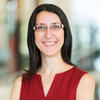
Louise St. Germain
19,424 PointsHi Kyla,
Step 1: Don't panic. You can do this! :-)
Sometimes the best way to start a tricky coding challenge is not with code at all. Try starting with pseudo-code, which is basically just writing out the logic in plain English. You can worry about how to turn it into Python code later, but if you can't explain how the program will work using normal language, that's probably where the problem is. Also, even if you don't quite know how the whole thing is going to work, writing down the bits you DO know will help you figure out exactly where the holes are and work on figuring those out, instead of having a mess of semi-ideas floating around in your head.
For this challenge, your pseudo-code might be something like this (though I am certainly not suggesting this is the only way, nor the best way - feel free to play around with whatever logic works for you):
# Take in a phrase that is a string.
# Make a list of which letters are vowels (since we will need to look for those in the phrase later).
# Turn the phrase that we were given into a list of its individual characters.
# Start a new list, empty for now, which is going to contain our output list of non-vowel characters.
# For each character in our original list of characters:
# Compare it to the list of vowels.
# If the character is not in the vowel list (i.e., is good):
# Add it to a list of non-vowel characters.
# Otherwise, do nothing (i.e., do not add it to the list of non-vowel characters).
# Once all the letters have been checked, turn the list of remaining (good) letters back into a single phrase (string).
# Return the string that has no vowels.
Once you have a program in pseudo-code, similar to the above, that works for you, then you can start filling it out with real code. In fact, the first line is already done in the challenge:
# Take in a phrase that is a string.
def disemvowel(word):
In this case, the "phrase" we talked about in pseudo-code is the parameter called word
, which the function disemvowel
is set up to receive. First line done!
Next up, make a list of vowels. So let's use a Python list (and we'll add it to the first bit we already had):
# Take in a phrase that is a string.
def disemvowel(word):
# Make a list of which letters are vowels (since we will need to look for those in the phrase later).
list_of_vowels = ["A", "a", "E", "e", "I", "i", "O", "o", "U", "u"]
That item is now done too! (Note that it's up to you to decide whether the list needs both uppercase and lowercase, as I've done, or whether you will just use one set.)
So literally just go through it line by line, until you have finished converting the English code to Python code.
Another tip is to test as you go when you're writing the Python code, instead of waiting until the end. (Try working on this in Workspaces before putting the final code into the challenge.) For example, when you do your line of code that splits the phrase into its individual characters, test it out to make sure it's doing what it's supposed to be doing (i.e., that what it's doing matches what you said in English it was supposed to do). By testing small chunks as you go, if something goes wrong, you only have to debug your newest code, rather than try to find the problem somewhere in your entire program.
I hope this helps to get you going! Let me know if you have any more questions, and good luck! You've got this!