Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial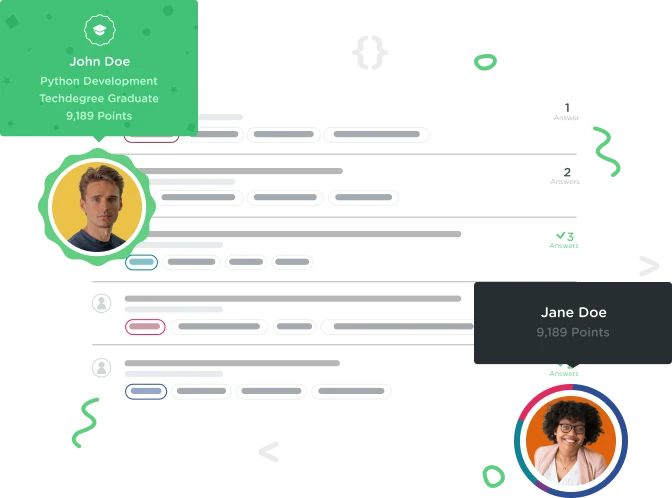
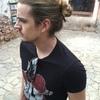
Juan Antonio Mejia Vazquez
903 Pointsdisemvowel
I don't know what is wrong with this code, could someone help me out?
def disemvowel(word):
vowels = ['a','e','i','o','u']
word = list(word)
for letter in word:
if letter in vowels:
word.remove(letter)
word = format("".join(word))
print("Your word without vowels is {}".format(word))
return word
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Juan,
Your code isn't going to work anytime you have consecutive vowels. You'll always miss the second vowel.
This is because you're modifying word
while you're iterating over it. When you remove one vowel, all the remaining letters move down one spot to occupy that empty spot. So you end up missing whichever letter came after the one you just removed.
Instead, you can iterate over a copy of word while you remove letters from word
for letter in word[:]:
I used slice notation to get a copy.

Torsten Lundahl
2,570 Points word = format("".join(word))
Theres is no need for the format(). Simply remove it and assign the join method directly to word.
word = "".join(word)

Torsten Lundahl
2,570 PointsAnd also! Your function isn't looking for uppercase vowels. Add uppercase vowels to the list to make sure you are removing those too.
vowels = ['a','e','i','o','u','A','E','I','O','U']
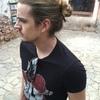
Juan Antonio Mejia Vazquez
903 PointsTorsten, I made the changes you mention but still there are some words that don't work like "antonio", it leaves: ntno. I used pycharm to debug the script but somehow it skips the last vowel.
Juan Antonio Mejia Vazquez
903 PointsJuan Antonio Mejia Vazquez
903 PointsThanks Jason, now the script works by removing all the vowels from the word, I don't quite undestand how the slice notation works yet, but I'm experimenting with it. Something weird is that I'm still not able to pass the code challenge, not even with the solution you gave me. I tested it in PyCharm and works.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsI'm not sure what your code looks like at this point but you can compare with the following:
This is mostly your code with the 2 necessary corrections. Looping over a copy of the word and accounting for uppercase vowels. As Torsten mentioned, the format() function isn't doing anything here but it will pass with it. I would take that out as well as the print statement. Extra things like that can sometimes cause your code not to pass.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsSorry about the suggestion to use slice notation. I didn't realize it wasn't covered until the next stage. It should make much more sense after covering the next stage.
I'll give you some hints on an alternative solution that I think is easier to understand and I think it only uses what you should know up to this point.
Here's some commented code. Each comment can be replaced with 1 line of code.
The idea behind this one is that you build up a new string consisting of all the letters in
word
that are not vowels.Juan Antonio Mejia Vazquez
903 PointsJuan Antonio Mejia Vazquez
903 PointsThanks Jason, that hint really helped. I finally passed the code challenge