Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial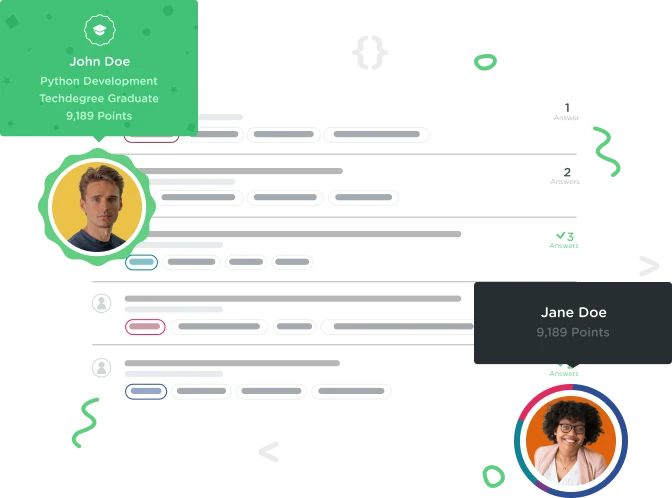
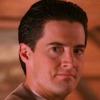
akoniti
1,410 PointsDisemvowel - So close!
I'll be honest, and admit that I initially gave up on this challenge during the Lists block of Python Basic, and moved on to Slices. Now that I've finished that (and gained additional skills and knowledge), I'm back to my old nemesis :)
I've very close to solving it. My code works great in every instance except when two vowels in a row are present in the input. In that case, it faithfully deletes the first vowel, but keeps the second vowel in the list.
I've tried and tested so many different approaches, and gone through the logic on a whiteboard multiple times, and am at a loss. There must be some aspect of iterating through (and simultaneously modifying) a list that I'm overlooking which is the cause. My thought is that it has something to do with the 'index' variable, and how I'm increasing it as my for loop iterates, but I can't crack it.
Any input would be much appreciated!
def disemvowel(word):
index = 0
vowels = ["a","e","i","o","u"]
mod_list = list(word)
for step in mod_list:
if step.lower() in vowels:
del mod_list[index]
index += 1
return ''.join(mod_list)
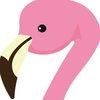
Dave StSomeWhere
19,870 PointsGlad you got it figured out. Just to point out that it can be simpler, you don't need the index stuff and could just use list.remove()
like:
def disemvowel(word):
vowels = ["a","e","i","o","u"]
mod_list = list(word)
for step in word:
if step.lower() in vowels:
mod_list.remove(step)
return ''.join(mod_list)
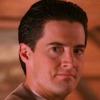
akoniti
1,410 PointsAh! Yes, that approach is simpler. I think I've just been so fixated on fiddling with specific indexes on this one, for so long, that I'm blind to other approaches :) Thanks again for your quick help, your guidance was expert.
1 Answer
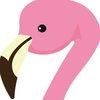
Dave StSomeWhere
19,870 PointsFirst, kudos for knowing what is wrong, that's the most important thing.
So, why do you want to iterate and simultaneously modify a list? In order to do that would involve some pain in the noodle index control.
Now, what other options do we have?
- You could always do a deep copy on the list and then iterate over the 1st list and modify the deep copy.
- You could also iterate over the original string which you called
word
and modify mod_list - this seems to me to be the easiest approach. Remember thatword
is a string, still iterable just immutable.
akoniti
1,410 Pointsakoniti
1,410 PointsYes! Thank you 🍹 Dave 🌴 StSomeWhere!
Your advice to 'Remember that
word
is a string, still iterable just immutable' was exactly what I needed. Simply iterating throughword
, and only moving the indexes that were not vowels intomod_list
did the trick. The only hitch was that I was left with trailing indexes not overwritten, but a finaldel mod_list[index:]
before thereturn
clears that up.EDIT
Thinking about it more,
mod_list
doesn't actually need to be created fromword
with this approach. I can just make it an empty list, and use.append
to add index values to it, which means I can eliminate thedel mod_list[index:]
step: