Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial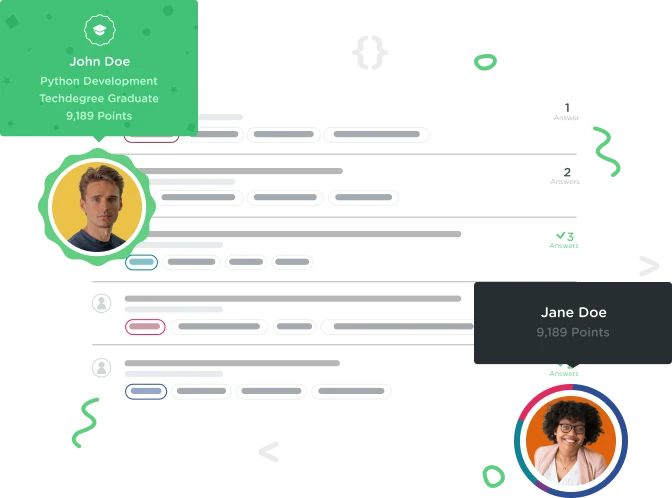

Alfonso Lopez
6,089 PointsDisemvowel: Uncertain why solution does not receive a pass.
I have tested the following in an iPython notebook and appears to work in different test cases for a single word. Can anyone tell me why the solution does not pass the requirement of removing all vowels from a given work (tested for upper and lower case).
def disemvowel(word):
word = list(word)
vowels = ["a", "e", "i", "o", "u", 'A', 'E', 'I', 'O', 'U']
x = True
for letter in word:
if letter in vowels:
while x == True:
try:
word.remove(letter)
except:
break
else:
continue
word = ''.join(word)
return word
2 Answers
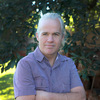
Christopher Shaw
Python Web Development Techdegree Graduate 58,248 PointsUnfortunately, you have to rethink your answer.
Test it with:
disemvowel('aeiouAEIOU')
and you will get back 'eoAIU'.
This is because, as it removes the first 'a', the list shifts left, so the next item is not 'e' as you are expecting, but 'i'.
Rather than iterate the word, iterate the vowels, then use word.remove(vowel) and this will remove all instances of that vowel from the word. Also, there is no need for the try, as there will be no exception if no letter is removed.
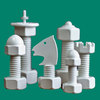
Steven Parker
229,744 PointsI bet you didn't test it on a word like "boat".
When you iterate on a sequence, and then modify the same sequence inside the loop, you can cause unexpected behavior such as having items skipped over.
Two ways to avoid this are to iterate on a copy of the sequence, or instead of modifying it in the loop, construct a new result instead.
Also, you dont need "x". You can just say "while True:
".

Alfonso Lopez
6,089 PointsThanks, I'll remember that for the while loop.
Here it is for "balloon": https://screencast.com/t/fOeMeACKch
You can see the result in the lower-left hand corner. It appears to work for this. However, "eoAIU" does return "oI" so I will investigate further.
Thanks again for the help!
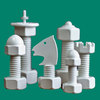
Steven Parker
229,744 PointsI changed my example word to "boat", I had suggested "baloon" without trying it first myself. But the principle is correct.
The quickest fix might be to use a slice to make a copy of the iterable:
for letter in word[:]:
Steven Parker
229,744 PointsSteven Parker
229,744 PointsBut doesn't remove act only on the first instance of a term?