Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial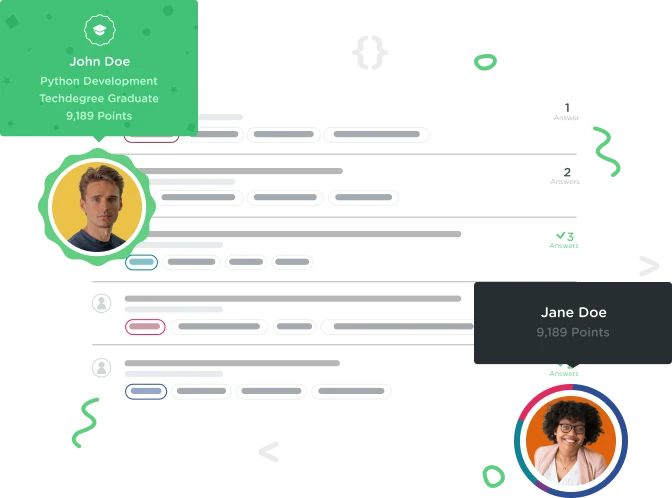
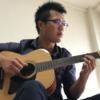
CHEN HAOMING
5,376 Pointsdisemvowel.py
The quiz is OK, I need you to finish writing a function for me. The function disemvowel takes a single word as a parameter and then returns that word at the end. I need you to make it so, inside of the function, all of the vowels ("a", "e", "i", "o", and "u") are removed from the word. Solve this however you want, it's totally up to you! Oh, be sure to look for both uppercase and lowercase vowels!
Here is my code. Can anybody help me to check out what happen with my code? I'm not sure where is not correct of my code. Thx, Chin.
vowels = ("a", "e", "i", "o", "u")
def disemvowel(word):
for letter in word:
if letter.lower() in vowels:
word.remove(letter)
else:
return word
vowels = ("a", "e", "i", "o", "u")
def disemvowel(word):
for letter in word:
if letter.lower() in vowels:
word.remove(letter)
return word
3 Answers
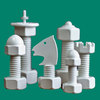
Steven Parker
230,274 PointsThe remove method is for lists, not strings.
You could convert your word into a list and then back into a string later. Or you could use a string method like "replace" instead.

Eric Biz
4,916 PointsMy answer works anywhere but workspace.
def disemvowel(word): word=word.lower() word=list(word) vowels=["a","e","i","o","u"] for vowel in vowels: while (vowel in word): word.remove(vowel) else: pass return word
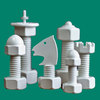
Steven Parker
230,274 PointsThe challenge wants you to remove the vowels without changing the other letters. But this code converts all the letters to lower case.
Also, when posting code, use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
 Or watch this video on code formatting.

Leon Silcott
27,583 PointsThere's probably a much better way of doing it, but below is the function I made. I hope it helps.
def disemvowel(word):
vowels_list = ["a", "e", "i", "o", "u"]
vowels_list_upper = [x.upper() for x in vowels_list]
new_word=[]
for letter in word:
if letter not in vowels_list:
if letter not in vowels_list_upper:
new_word.append(letter)
return "{}".format(''.join(new_word))
CHEN HAOMING
5,376 PointsCHEN HAOMING
5,376 PointsThank you dear Steven I have tried this.
it worked.
but if I tried this one
if I add this
My code will not work.
Would you like to tell me why.My friend.
Best regards, Chen.
Steven Parker
230,274 PointsSteven Parker
230,274 PointsWell, one approach to this challenge is to create a new string of the characters you want to keep. You are doing this with your
result
. Another approach is to remove things you don't want to keep, but you don' t need to do this if you're doing the first approach.Also, modifying an iterable inside the loop where it is used can cause problems, like skipped items. That's one reason to use the first approach. When you add that else, you create those problems which you otherwise have already avoided.
CHEN HAOMING
5,376 PointsCHEN HAOMING
5,376 PointsThank for your ez understanding explanation. Thank you.