Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial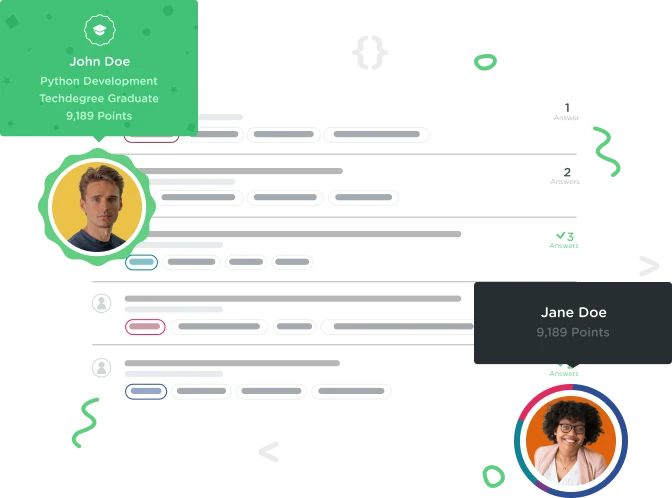

Sabaa Talal
Courses Plus Student 733 Pointsdisemvowel.py
hello, so this is the problem in hand i could not figure out how to start solving it. can you give me some tips and advice on how to start solving it? this is the question (OK, I need you to finish writing a function for me. The function disemvowel takes a single word as a parameter and then returns that word at the end. I need you to make it so, inside of the function, all of the vowels ("a", "e", "i", "o", and "u") are removed from the word. Solve this however you want, it's totally up to you! Oh, be sure to look for both uppercase and lowercase vowels!) thank you
def disemvowel(word):
return word
1 Answer
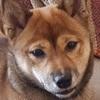
Katie Wood
19,141 PointsEdit for step-by-step detail
So the challenge wants us to take all of the vowels out of the word that is passed in. We know we need to check against a list of vowels, so let's make that list first:
def disemvowel(word):
vowels = ['a','e','i','o','u']
return word
Now, we know we also need a list that contains each letter of our word:
def disemvowel(word):
vowels = ['a','e','i','o','u']
letters = list(word)
return word
Now, we know we need to check each letter in the word and remove it if it's a vowel. Let's start by beginning a loop that will go through the word:
def disemvowel(word):
vowels = ['a','e','i','o','u']
letters = list(word)
for letter in word:
return word
So, for each letter we need to check to see if that letter appears in the vowel list, and remove it if it does. We also need it to not be case-sensitive, so we're going to make each letter lowercase before we compare. That looks like this:
def disemvowel(word):
vowels = ['a','e','i','o','u']
letters = list(word)
for letter in word:
if letter.lower() in vowels:
letters.remove(letter)
return word
We're almost there, but we don't actually want to return 'word' - that won't get us anywhere. We want to return the remaining letter in the letters list - that was where we were removing vowels from. So, let's return a join() of those instead:
def disemvowel(word):
vowels = ['a','e','i','o','u']
letters = list(word)
for letter in word:
if letter.lower() in vowels:
letters.remove(letter)
return ''.join(letters)
And there we go - we're done!
Hope this helps!
Sabaa Talal
Courses Plus Student 733 PointsSabaa Talal
Courses Plus Student 733 Pointsthank you for your help, but i did not understand what you said to me, can you show me what does it mean.
Katie Wood
19,141 PointsKatie Wood
19,141 PointsSure, I'll go through it step by step. I'll just update my answer, so it's easier to find if you ever want to refer back to it - it'll basically be the same thing but with more detail. Stand by!