Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial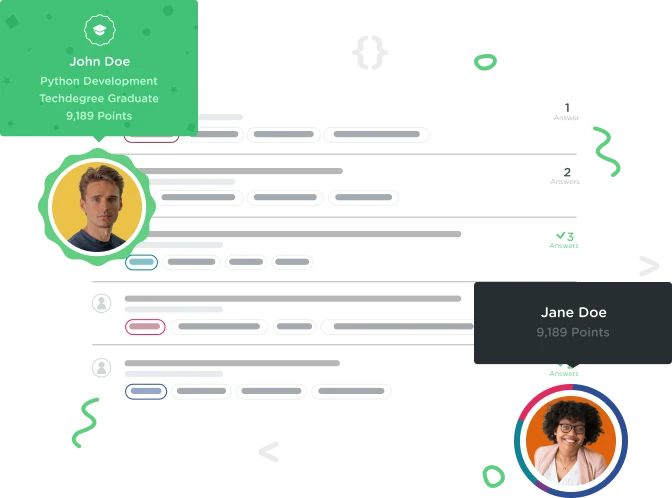

Lucas Garcia
6,529 Pointsdisemvowel.py challenge
I am getting a syntax error. Also, do you think this code will solve the challenge?
def disemvowel(word): list(word) while True: if 'a' is in word.lower(): word.remove('a') elif 'e' is in word.lower(): word.remove('e') elif 'i' is in word.lower(): word.remove('i') elif 'o' is in word.lower(): word.remove('o') else 'u' is in word.lower(): word.remove('u') break
return word
def disemvowel(word):
list(word)
while True:
if 'a' is in word.lower():
word.remove('a')
elif 'e' is in word.lower():
word.remove('e')
elif 'i' is in word.lower():
word.remove('i')
elif 'o' is in word.lower():
word.remove('o')
else 'u' is in word.lower():
word.remove('u')
break
return word
2 Answers

salman khan
1,283 PointsHere's my solution for the challenge..
def disemvowel(word):
vowels = ("a", "e", "i", "o","u", "A", "E", "I", "O", "U")
for vowel in word:
if vowel in vowels:
try:
word = word.replace(vowel, "")
except ValueError:
pass
print(word)
return word
wr=input("enter a word ")
disemvowel(wr)
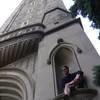
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsThere are several problems with your code. First, here is my solution:
def disemvowel(word):
word = list(word)
for key in word.copy():
if key.lower() == 'a':
word.remove(key)
elif key.lower() == 'e':
word.remove(key)
elif key.lower() == 'i':
word.remove(key)
elif key.lower() == 'o':
word.remove(key)
elif key.lower() == 'u':
word.remove(key)
return word
Problem 1: list(word)
You are calling the list
method on the argument to the function without assigning the return value to a variable. The list
method returns an iterable as a list, but it doesn't convert the iterable to a list in place. You have to save it to a variable, which is why you will see in my solution, I use word = list(word)
.
Problem 2: is in...
Using "is in" is not valid syntax in Python. You're being a bit too human for the computer. All the computer requires, when you speak to it in Python, is if x in y: do z
. If you try to use if x is in y...
it will throw an error.
Problem 3: Not iterating over a copy
When you are simultaneously iterating over a list and making changes to that list, like removing elements, you will need to iterate over a copy()
of that list, but change the list. This is because iterating over a list and also making changes to it at the same time causes strange results, at least to our human brains. Instead, when you are going to iterate over a list and also make changes to it, do it the way I have done it in my example. Use the following format:
for key in list.copy():
if key == x:
list.remove(x)
Notice that I am iterating over a copy of the list, and removing from the original. Remembering this will save you a world of frustration.
Problem 4: Using a while loop When iterating over a list or another iterable, in most cases you will want to use a for loop, not a while loop. While loops are generally used to do the same thing over and over until a certain condition becomes true. But when you are doing something to an iterable data structure like a list, you just want the process to keep on going until it reaches the end of the data structure. In a for loop, you set a variable, and at each iteration of the loop, the variable you set gets assigned to the next item in the list. This allows you to grab ahold of each piece of data in the list and check it for any condition you set and then do something with it. This is harder to accomplish with a while loop. So remember: if you want to keep doing the same process over and over again, use a while loop. But if you want to check a bunch of pieces of data or do something to a bunch of pieces of data, use a for loop.
Good luck!

Lucas Garcia
6,529 PointsWow Michael.
Thank you so much for the excellent reply. You really cleared a lot of questions up that I had. How to I convert the list back to a string? Your code works but the challenge doesn't like it because the answer is printed out as a list instead of a string. I loved how you really broke down all the mistakes with the number topics so it was clear and easy to understand. Thank you Michael!
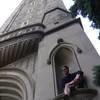
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsI think to convert an iterable to a string you just use the str()
method. I suggest getting into the habit of googling questions and reading documentation. I know documentation is technical and difficult to understand at first but over time it gets easier and it is there to help you. Glad my answer helped!
Lucas Garcia
6,529 PointsLucas Garcia
6,529 PointsHi Salman,
Thank you so much for the solution. Your code is very clean and works well. Thank you for taking the time to help me. Have a great day!
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsThis is an elegant solution. I like it.
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsYou could have written less code by leaving the uppercase letters out of the tuple and just using the ´lower()´ method in the conditional. But still, I like your approach.