Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial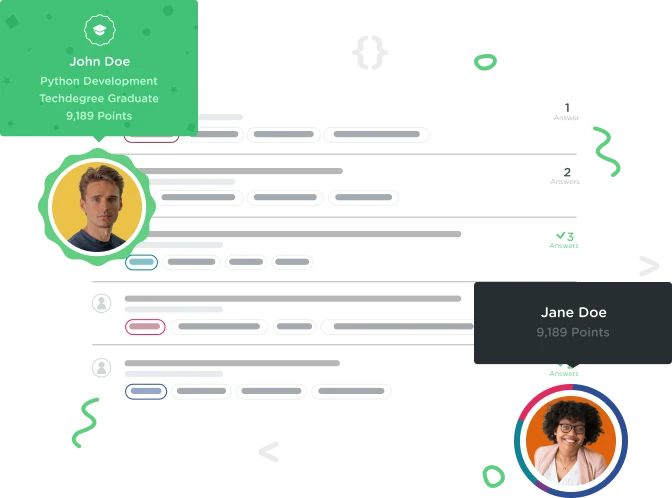

arielp
3,516 Pointsdisemvowels.py why pycharm is happy with my code and still can't pass the challenge..?
Here is my solucion for this challange:
for some reason still can't pass the challenge and continue the course, pycharm seems to be happy with this code... thanks Ari
text = input('Enter a word: ')
Word = list(text)
def desimvowels(word):
vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
for vowel in vowels:
if vowel in word:
word.remove(vowel)
print(word)
return word
desimvowels(Word)
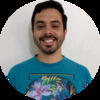
Ewerton Luna
Full Stack JavaScript Techdegree Graduate 24,031 PointsThere are some things in your code you should change. You got the right idea, but your code has some logical errors. First off, your for loop is iterating through the vowels list. The problem is that, for exemple, if your word has two letters 'a', it will only remove one of them, since the for loop goes through each element once. It would be better if you had your for loop iterating through the word variable.
I'm gonna write a different version based on your code:
def desimvowels(word):
word = list(word) # Reassigned the word varible into a list version of itself.
vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
for letter in word.copy(): # If you are going to modify a list when iterating through it, you whould iterate through a copy of it
if letter in vowels:
word.remove(letter)
return "".join(word) # You should return a string, not a list.
I hope this can help! Feel free to ask any further questions. I'm glad to help.
4 Answers
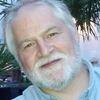
Jeff Muday
Treehouse Moderator 28,723 PointsI see you are on the right track, let's build on that. There are a bunch of ways to solve this challenge, but you are definitely close to a solution!
Challenges don't allow for extra debugging input/output so we have to get rid of the input and calling function.
The Challenge is expecting "word" argument to be a string, and not a list. This requires using the "replace" method of the string-- strings don't have a remove() method.
because strings are immutable, we need to assign word to the value returned from word.replace() method.
Good luck with your Python journey, it's not easy, but it is worthwhile!
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
for vowel in vowels:
if vowel in word:
word = word.replace(vowel,'') # here is the main change required.
return word

arielp
3,516 PointsI see, so the page would only accept one way of input as the right answer?
thanks for your help, very clear.!

arielp
3,516 Pointsthanks a lot guys...!
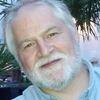
Jeff Muday
Treehouse Moderator 28,723 PointsThe way code-challenges work, it only tests specifically a function, class method, or code-segment output. The "grader" for the code-challenges are similar to Python unittest.
https://docs.python.org/2/library/unittest.html
But I definitely like your choice of PyCharm for a development/testing environment (especially the PyCharm EDU version is great, especially their "code-linter"). I use WingWare Pro, but the Personal edition is free (http://wingware.com/downloads/wing-personal) has a slightly better debugger than PyCharm.

arielp
3,516 Pointsmany thanks for all that info! Jeff, much appreciated I'm going to give wing a go, I think I can get an annual pro licence via UNI.
thanks again.

arielp
3,516 PointsHey Jeff, You were spot on about the debugging power of wing! I haven't played much with it yet but my first impression is that it seems to flag more things and in a better way! very useful for starters. thanks a lot again.!!

arielp
3,516 Pointsthank you all guys.! I finally got the challenge happy with this code:
Word = 'aaaaRDaaRddeeeeeeeiiiiidHGJiiiiiioooroooooooRouuuuuuruuAAAArAAEEEErIIIROOOOUUU'
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
for vowel in vowels:
if vowel in word:
word = word.replace(vowel, '')
print(word)
return word
disemvowel(Word)
pet
10,908 Pointspet
10,908 PointsThis is a more proper version of your code.
Odd both this and yours work in workspace. Also you misspelled desimvowels Kenneth wanted disemvowel. Hope this helps. :)