Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial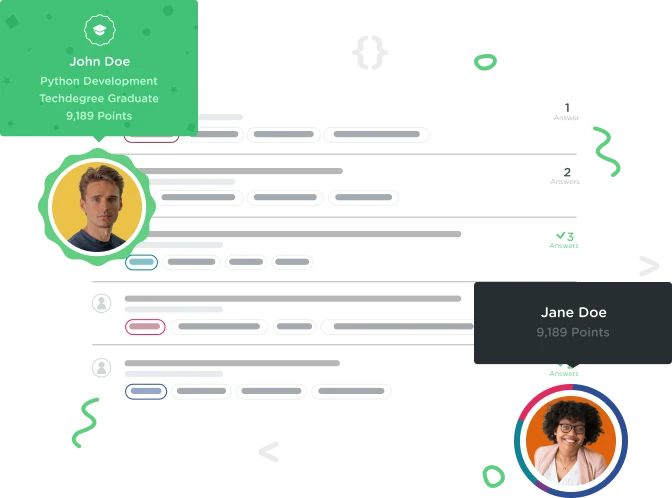
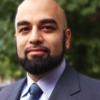
Hamed Ali
5,626 PointsDisplay a modal. There should be a button to close the modal. Anyone know why dismiss button isn't working?
Here is the error message:
The last element in the modal is the placeholder, not the button. Did you use append()
to append the button to the modal?
//Create the Modal
var $modal = $("<div id='modal'></div>");
//Create a placeholder for text in the modal
var $placeHolder = $("<p id='placeHolder'></p>");
$modal.append($placeHolder);
//Create a button to dismiss modal and add it to modal
var $dismissButton = $("<button>Dismiss</button>");
//Hide modal when button is pressed
$dismissButton.click(function(){
$modal.hide(300);
});
$("body").append($modal);
//A function to show a modal
function displayModal(message) {
$placeHolder.text(message);
$modal.show();
}
//Show an example modal
displayModal("Hello World!");
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
<title>Modal Example</title>
</head>
<body>
<h1>Modal Example Page</h1>
<script src="//code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
3 Answers
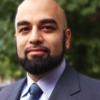
Hamed Ali
5,626 Pointswell I had written:
$("body").append($dismissButton);
and placed it right after the $("body").append($modal); .... This should have worked - and I tried to place it in a few different place but no luck. Im probably doing a very small silly thing.
Also tried $dismissButton.show(); in the displayModal function but also no luck. (That would not have worked anyways.
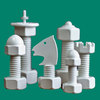
Steven Parker
231,275 PointsIt's not the document body that the button should be added to.
The challenge says the button should be added to the modal ($modal).
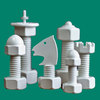
Steven Parker
231,275 PointsYou say this worked:
$($modal).append($dismissButton);//HERE IS THE CODE
But since $modal is already a jQuery object, you really only need this:
$modal.append($dismissButton);
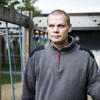
mikkel pohjola
1,966 Pointsi didt it like this and that work
<p>//Create the Modal
var $modal = $("<div id='modal'></div>");
//Create a placeholder for text in the modal
var $placeHolder = $("<p id='placeHolder'></p>");
$modal.append($placeHolder);
//Create a button to dismiss modal and add it to modal
var $dismissButton = $("<button>Dismiss</button>");
//Hide modal when button is pressed
$dismissButton.click(function(){
$modal.hide(300);
});
$($modal).append($dismissButton);//HERE IS THE CODE
$("body").append($modal);
//A function to show a modal
function displayModal(message) {
$placeHolder.text(message);
$modal.show();
}
//Show an example modal
displayModal("Hello World!");</p>
```
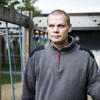
mikkel pohjola
1,966 Pointsit makes sense, even though it did the other: D