Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial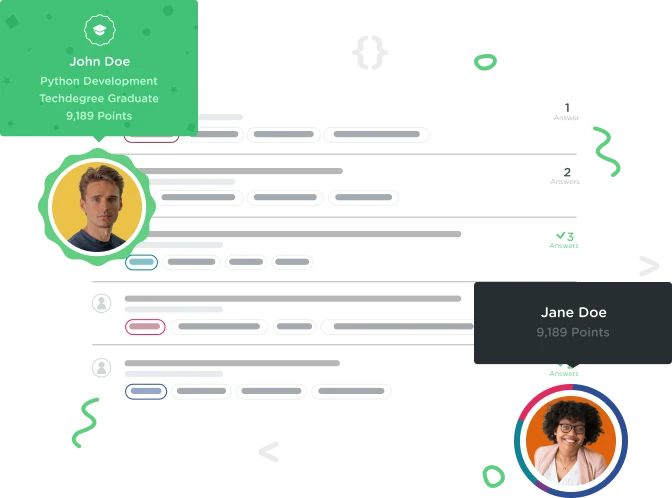

Erik L
Full Stack JavaScript Techdegree Graduate 19,470 PointsDisplay images using javascript link of my project: https://github.com/goldenwarrior117/FSJS-techdegree-project-1
Hello I am trying to display several images within each of my arrays, but the code I typed is not working, can anyone help?
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Random Quotes</title>
<link href='https://fonts.googleapis.com/css?family=Playfair+Display:400,400italic,700,700italic' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/normalize.css">
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<div class="container">
<div id="quote-box">
<p class="quote">Every great developer you know got there by solving problems they were unqualified to solve until they actually did it.</p>
<p class="source">Patrick McKenzie<span class="citation">Twitter</span><span class="year">2016</span></p>
</div>
<button id="loadQuote">Show another quote</button>
</div>
<script src="js/script.js"></script>
<script>
setInterval(function() {
var randomColor = Math.round( Math.random() * 255 );
var element = document.getElementById("loadQuote");
},2000);
</script>
</body>
</html>
// FSJS - Random Quote Generator
/* I created an array[] of quote objects{} and I named it (quotes)
*/
var quotes = [
{ quote: "The biggest risk is not taking any risk... In a world that's changing really quickly, the only strategy that is guaranteed to fail is not taking risks.",
source: 'Mark Zuckerberg',
citation: "Facebook's Mark Zuckerberg -- Insights For Entrepreneurs",
year: '2011' ,
occupation: '--Former Programmer/Businessman',
Image: 'images/MarkZ.jpg',
},
{
quote: 'Not everything that can be counted counts, and not everything that counts can be counted.',
source: 'William Bruce Cameron ',
citation: 'Informal Sociology: A Casual Introduction to Sociological Thinking',
year: '1963' ,
occupation:'--Book Author',
Image: 'images/BruceC.jpg',
},
{
quote: 'Life is 10% what happens to you and 90% how you react to it.',
source: 'Charles R. Swindoll',
citation: 'https://www.brainyquote.com/quotes/charles_r_swindoll_388332',
year: '2011' ,
occupation: '--Pastor/Author',
Image: 'images/CharlesR.jpg',
},
{
quote: 'Our greatest weakness lies in giving up. The most certain way to succeed is always to try just one more time',
source: 'Thomas A. Edison',
citation: 'https://www.brainyquote.com/quotes/thomas_a_edison_149049',
year: '1931' ,
occupation: '--Inventor/Businessman',
Image: 'images/ThomasH.jpg',
},
{
quote: "I'd rather attempt to do something great and fail than to attempt to do nothing and succeed.",
source: 'Robert H. Schuller',
citation: 'https://www.brainyquote.com/quotes/robert_h_schuller_155998',
year: '2015' ,
occupation: '--Pastor/Motivational Speaker',
Image: 'images/RobertH.jpg',
}
];
/*
choose and then return a random quote
object from the array
*/
function getRandomQuote(array) {
var quoteIndex = Math.floor( Math.random() * (quotes.length));
for (var i = 0; i < array.length; i++) {
var randomQuote = array[quoteIndex];
}
return randomQuote;
}
// I created a second function and gave it (printQuote) as its name.
function printQuote() {
var message = ""; // This is the message variable with empty strings
var result = getRandomQuote(quotes);
message = "<p class='quote'>" + result.quote + "</p>";
message += "<p class='source'>" + result.source;
message += "<span class='citation'>" + result.citation + "</span>";
message += "<span class='year'>" + result.year + "</span>";
message += "<span class='occupation'>" + result.occupation + "</span>";
message += "<span class='Image'>" + result.Image + "</span>";
message += "</p>";
document.getElementById('quote-box').innerHTML = message;
}
printQuote();
// This event listener will respond to "Show another quote" button clicks
// when user clicks anywhere on the button, the "printQuote" function is called
document.getElementById('loadQuote').addEventListener("click", printQuote, false);
1 Answer
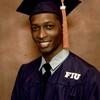
Dane Parchment
Treehouse Moderator 11,077 PointsOkay so you've got quite a few things wrong with this code, but don't let it discourage you, let me walk you through your problems.
First and foremost, let's take a look at your getRandomQuote(array)
function. It is poorly implemented, so let's first take a look at what you are doing wrong, which is 3 important things.
- You are creating an unnecessary for loop, you don't need to loop through the array, you have already generated a random number, so just return the array[randomNumber]. If you see that you are looping through code and not using any of the loops pointers (i in your case), then the loop is not necessary.
- You are declaring the variable within the for loop, meaning it won't be accessible outside of the for loop, meaning you can't return that variable, you will get an undefined, as the variable hasn't been defined outside the function. This is a scoping issue.
- You aren't using the parameter that you setup for the function. Your function takes a parameter called
array
, but instead of using that parameter to get it's length you are using the instance variable you defined earlier code. This may break code later if you plan to use an array with a length greater or less than your quote array. Basically, per the way you implemented this function...the parameter isn't necessary at all, it isn't being used properly and will lead to errors.
So with the mistakes there known, let's fix that function to be more efficient!
function getRandomQuote(array) {
return array[Math.floor(Math.random() * array.length)];
}
With that done, you are one step closer to solving your problem! Now let's take a look at that function printQuote
Basically you are good here. You made one mistake though, and that is if you want to display the images, you aren't using any img
tags. Remember those from html? <img src="path to image">
So basically we are just going to change the <span class=Image>
into an actual image tag. So your function should look like this:
function printQuote() {
var message = ""; // This is the message variable with empty strings
var result = getRandomQuote(quotes);
message = "<p class='quote'>" + result.quote + "</p>";
message += "<p class='source'>" + result.source;
message += "<span class='citation'>" + result.citation + "</span>";
message += "<span class='year'>" + result.year + "</span>";
message += "<span class='occupation'>" + result.occupation + "</span>";
message += "<img src=" + result.Image +">";
message += "</p>";
document.getElementById('quote-box').innerHTML = message;
}
So in total, your code should look like so!
var quotes = [
{ quote: "The biggest risk is not taking any risk... In a world that's changing really quickly, the only strategy that is guaranteed to fail is not taking risks.",
source: 'Mark Zuckerberg',
citation: "Facebook's Mark Zuckerberg -- Insights For Entrepreneurs",
year: '2011' ,
occupation: '--Former Programmer/Businessman',
Image: 'https://images.unsplash.com/photo-1534990874943-dceb856eff5b?ixlib=rb-0.3.5&s=f7d200bc56f5db5ec0b7303fb7e16b4e&auto=format&fit=crop&w=634&q=80',
},
{
quote: 'Not everything that can be counted counts, and not everything that counts can be counted.',
source: 'William Bruce Cameron ',
citation: 'Informal Sociology: A Casual Introduction to Sociological Thinking',
year: '1963' ,
occupation:'--Book Author',
Image: 'https://images.unsplash.com/photo-1535086181678-5a5c4d23aa7d?ixlib=rb-0.3.5&s=7f5caa98714366dda89492285feff243&auto=format&fit=crop&w=1489&q=80',
},
{
quote: 'Life is 10% what happens to you and 90% how you react to it.',
source: 'Charles R. Swindoll',
citation: 'https://www.brainyquote.com/quotes/charles_r_swindoll_388332',
year: '2011' ,
occupation: '--Pastor/Author',
Image: 'https://images.unsplash.com/photo-1535112512675-718c648e23a7?ixlib=rb-0.3.5&ixid=eyJhcHBfaWQiOjEyMDd9&s=791228dedd138dc1c359f500fd8c7737&auto=format&fit=crop&w=1979&q=80',
},
{
quote: 'Our greatest weakness lies in giving up. The most certain way to succeed is always to try just one more time',
source: 'Thomas A. Edison',
citation: 'https://www.brainyquote.com/quotes/thomas_a_edison_149049',
year: '1931' ,
occupation: '--Inventor/Businessman',
Image: 'https://images.unsplash.com/photo-1535133171663-a4bd711d1a8a?ixlib=rb-0.3.5&s=5ab0efe38116351d3bfc257ca1b2ffec&auto=format&fit=crop&w=2050&q=80',
},
{
quote: "I'd rather attempt to do something great and fail than to attempt to do nothing and succeed.",
source: 'Robert H. Schuller',
citation: 'https://www.brainyquote.com/quotes/robert_h_schuller_155998',
year: '2015' ,
occupation: '--Pastor/Motivational Speaker',
Image: 'https://images.unsplash.com/photo-1535124827275-dd7536f8db5a?ixlib=rb-0.3.5&s=91119eaffe7c709c04eb0c2a00556a3f&auto=format&fit=crop&w=2134&q=80',
}
];
function getRandomQuote(array) {
return array[Math.floor(Math.random() * array.length)];
}
function printQuote() {
var message = ""; // This is the message variable with empty strings
var result = getRandomQuote(quotes);
message = "<p class='quote'>" + result.quote + "</p>";
message += "<p class='source'>" + result.source;
message += "<span class='citation'>" + result.citation + "</span>";
message += "<span class='year'>" + result.year + "</span>";
message += "<span class='occupation'>" + result.occupation + "</span>";
message += "<img src=" + result.Image +">";
message += "</p>";
document.getElementById('quote-box').innerHTML = message;
}
var button = document.getElementById('quote-clicker');
button.addEventListener('click', function() {
console.log("Hi");
printQuote();
});
(Ignore the image links, I used a website to get them, you can use your own links instead)
Here is the link to a CodePen I created with this code showing it working

Erik L
Full Stack JavaScript Techdegree Graduate 19,470 PointsThanks so much, you have no idea how much your feedback helped!, one more question though, I would like to make my images centered and circular, I am aware that I have to use border-radius: 50%;
, however in this situation my img src is located on the javascript file and not the html file. Are we able to add css code to a js file?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsIt would be easier to analyze code using images with the complete project. You could make a snapshot (note: not "screenshot") of your workspace and post the link to it here.