Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial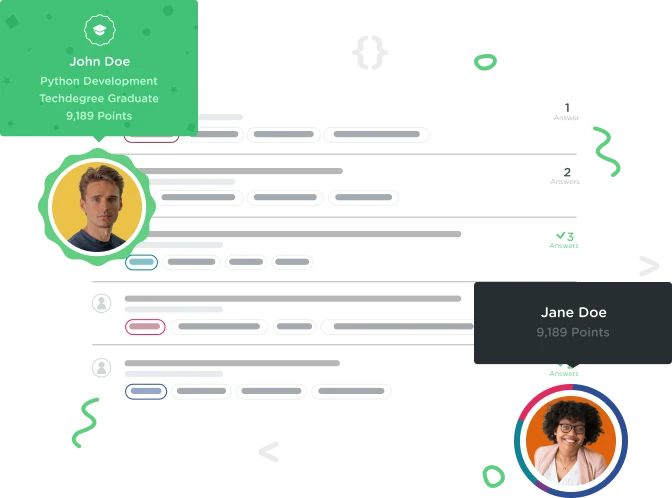

Milan Tailor
5,132 PointsDisplaying images in grid - Empty grid
I can see the images in the file system, but they don't seem to be showing. I've double checked and I think my code is right, what have I buggered up?
Any help appreciated.
ImageGridFragment
public class ImageGridFragment extends Fragment {
private GridView mGridView;
private GridViewAdapter mGridAdapter;
public static int RESULT_LOAD_IMAGE = 1000;
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_image_grid, container, false);
mGridView = (GridView) rootView.findViewById(R.id.gridView);
mGridAdapter = new GridViewAdapter(this.getActivity(), R.layout.view_grid, extractFiles());
mGridView.setAdapter(mGridAdapter);
mGridView.setOnItemClickListener(mOnItemClickListener);
mGridView.setOnItemLongClickListener(mOnItemLongClickListener);
this.setHasOptionsMenu(true);
return rootView;
}
@Override
public void onCreateOptionsMenu(Menu menu, MenuInflater inflater) {
inflater.inflate(R.menu.image_list, menu);
super.onCreateOptionsMenu(menu, inflater);
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
if(item.getItemId() == R.id.import_action) {
Intent intent = new Intent(Intent.ACTION_PICK, MediaStore.Images.Media.EXTERNAL_CONTENT_URI);
startActivityForResult(intent, RESULT_LOAD_IMAGE);
} else {
if (item.getItemId() == R.id.settings_action) {
Intent intent = new Intent(this.getActivity(), MemeSettingsActivity.class);
startActivity(intent);
}
}
return super.onOptionsItemSelected(item);
}
private ArrayList extractFiles() {
final ArrayList imageItems = new ArrayList();
File [] filteredFiles = FileUtilities.listFiles(this.getActivity());
for(File filteredFile : filteredFiles) {
Bitmap bitmap = BitmapFactory.decodeFile(filteredFile.getAbsolutePath());
ImageGridItem item = new ImageGridItem(bitmap, filteredFile.getName(),
filteredFile.getAbsolutePath());
imageItems.add(item);
}
return imageItems;
}
private void resetGridAdapter() {
mGridAdapter = new GridViewAdapter(this.getActivity(), R.layout.view_grid, extractFiles());
mGridView.setAdapter(mGridAdapter);
}
protected AdapterView.OnItemClickListener mOnItemClickListener = new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) {
ImageGridItem imageGridItem = (ImageGridItem) adapterView.getAdapter().getItem(i);
Intent intent = new Intent(ImageGridFragment.this.getActivity(), CreateMemeActivity.class);
intent.putExtra(CreateMemeActivity.EXTRA_IMAGE_FILE_PATH, imageGridItem.getFullPath());
Log.d("FILE:", imageGridItem.getFullPath());
ImageGridFragment.this.getActivity().startActivity(intent);
}
};
protected AdapterView.OnItemLongClickListener mOnItemLongClickListener = new AdapterView.OnItemLongClickListener() {
@Override
public boolean onItemLongClick(AdapterView<?> adapterView, View view, int i, long l) {
final ImageGridItem imageGridItem = (ImageGridItem) adapterView.getAdapter().getItem(i);
AlertDialog.Builder builder = new AlertDialog.Builder(ImageGridFragment.this.getActivity());
builder.setTitle(R.string.dialog_confirm)
.setMessage(R.string.dialog_message_delete_file)
.setPositiveButton(android.R.string.yes, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
File fileToDelete = new File(imageGridItem.getFullPath());
boolean deleted = fileToDelete.delete();
if (deleted) {
ImageGridFragment.this.resetGridAdapter();
}
}
})
.setNegativeButton(android.R.string.no, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
}
});
builder.create().show();
return true;
}
};
}
FileUtilities Class
public class FileUtilities {
public static void saveAssetImage(Context context, String assetName) {
File fileDirectory = context.getFilesDir();
File fileToWrite = new File(fileDirectory, assetName);
AssetManager assetManager = context.getAssets();
try {
InputStream in = assetManager.open(assetName);
FileOutputStream out = new FileOutputStream(fileToWrite);
copyFile(in, out);
in.close();
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
private static void copyFile(InputStream in, OutputStream out) throws IOException {
byte[] buffer = new byte[1024];
int read;
while((read = in.read(buffer)) != -1){
out.write(buffer,0 , read);
}
}
public static File [] listFiles (Context context) {
File fileDirectory = context.getFilesDir();
File [] filteredFiles = fileDirectory.listFiles(new FileFilter() {
@Override
public boolean accept(File file) {
if(file.getAbsolutePath().contains(".jpeg")) {
return true;
}
else {
return false;
}
}
});
return filteredFiles;
}
public static Uri saveImageForSharing(Context context, Bitmap bitmap, String assetName) {
File fileToWrite = new File(Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES), assetName);
try {
FileOutputStream outputStream = new FileOutputStream(fileToWrite);
bitmap.compress(Bitmap.CompressFormat.JPEG, 100, outputStream);
outputStream.flush();
outputStream.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
return Uri.fromFile(fileToWrite);
}
}
public static void saveImage(Context context, Bitmap bitmap, String name) {
File fileDirectory = context.getFilesDir();
File fileToWrite = new File(fileDirectory, name);
try {
FileOutputStream outputStream = new FileOutputStream(fileToWrite);
bitmap.compress(Bitmap.CompressFormat.JPEG, 100, outputStream);
outputStream.flush();
outputStream.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
1 Answer

Jun Kakeno
iOS Development with Swift Techdegree Graduate 24,842 PointsI have the same issue but I can't find the solution for this anywhere. Please help.