Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial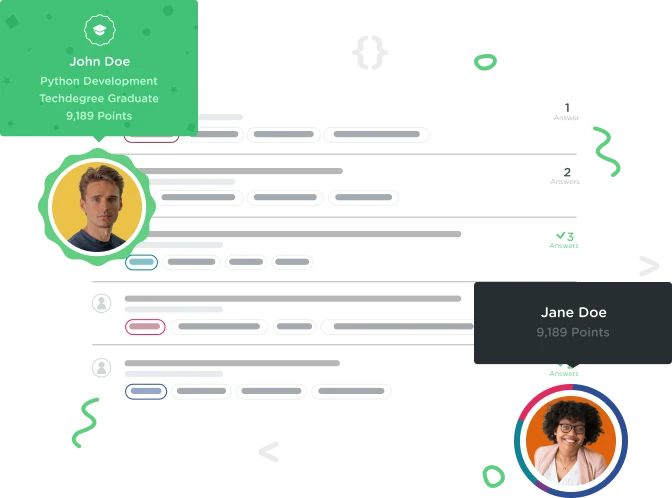

AUTIF KAMAL
11,692 PointsDisplaying Search Results via Spotify API
I'm trying to learn how to make JSON requests. Kind of struggling with it. Specifically, I'm trying to access the Spotify API and display search results (i.e. thumbnails of albums) based on user input. This is what I have so far. Please tell me what I'm doing wrong or missing. Thanks.
HTML
<!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script> <script src="script.js"></script> <link rel="stylesheet" type="text/css" href="styles.css">
</head>
<body>
<div class="top">
<h1>Spotify Song Search</h1>
<form>
<input type="search" placeholder="Search for a album or band" id="song">
<button type="submit" id="search">Search</button>
</form>
</div>
<div id="music">
</div>
</body>
</html>
JavaScript/JQuery
$(document).ready(function() {
$('form').submit(function(evt) {
evt.preventDefault();
var $searchField = $('#search');
var spotifyAPI = "https://api.spotify.com/v1/search" // needs end point
var albums = $searchField.val();// Specifies type of user info to be retrieved and sent to Spotify API
var spotifyOptions = {
"type": "album",
"q": "artist",
"limit": "limit"
}; // Requests data from spotify API. Must be a JavaScript Object
function displayAlbums(data){
var albumHTML = '<ul>'; // Opening ul tag to hold a single album result
$.each(data.items, function(i, album){
albumHTML += '<div class="album" album-id="' + index + '">';
albumHTML += '<li>'; // You may need to use bootstrap to style array items correctly
albumHTML += '<a href="#">';
albumHTML += '<img src="' + album.images[0].url + '" alt="' + album.artists[1].name + '">';
albumHTML += '</a>';
albumHTML += '</div>';
return albumHTML;
}
albumHTML += '</ul>';
$('#music').html(albumHTML);
});
}
$.getJSON(spotifyAPI, spotifyOptions, displayAlbums); // Currently, these are only placeholders. The variables inserted as arguments have not been defined as variables
});
});
Thanks for your time.
1 Answer

Seth Kroger
56,413 Points- Look at the data you're getting back from the API. Make sure the data objects are nested and named the way you think they are. I believe you want
data.albums.items
notdata.items
- Use DevTools and pay attention to error messages in the Console.
- You have mismatching braces and parentheses in displayAlbums() that are undoubtedly causing errors.
- You open an
<li>
but never close it. - You're pulling out the name of the 2nd artist listed, but most albums would only have one artist.
AUTIF KAMAL
11,692 PointsAUTIF KAMAL
11,692 PointsThanks for your advice. I'll make the corrections that you pointed out.
AUTIF KAMAL
11,692 PointsAUTIF KAMAL
11,692 PointsHTML
<!DOCTYPE html>
<html>
</html>
JavaScript
$(document).ready(function() {
So, I've been looking at the error messages in the Console. Whatever is entered in the search field is being passed through, so that's good. But, I'm getting a 400 error. Further guidance would be appreciated. Thanks again for your help/ advice.