Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial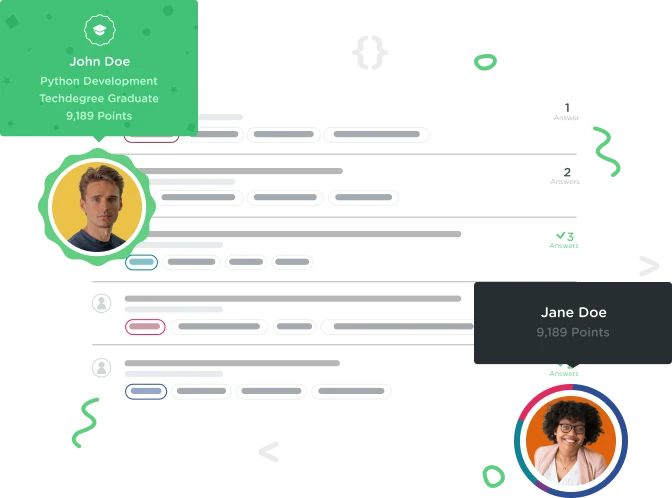

Richard Levy
554 PointsDistinguishing parameter labels, names and values
The function first appears in the form: func calculateArea(height: Int, width: Int) -> Int { return height * width } Applying Amit's opening explanation of parameters label and names, it seems to me that here the terms "height" and "width" are parameter labels and "Int" is the parameter value.
But when Amit edits the function he seems to imply that he is labelling the parameters, using labels which are the same as the existing local parameter names. The function then appears as: func calculateArea(height height: Int, width width: Int) -> Int { return height * width }
Does this mean that in the first version of the function the parameters were named but not labelled? And that in the second version of the function the parameters take the form: (label name: value, label name: value)?
Can you please help me understand the distinction between parameter labels and parameter names?
Thanks
3 Answers

Chris Shaw
26,676 PointsHi Richard,
All this terminology can be confusing right? Don't worry as Swift as many other upcoming iOS developers have trouble understanding the concept of parameter labels but it's actually quite simple. Let's break this down into three parts.
1. What a basic function looks like, how does it behave?
I'll use the calculateArea
function as it's a good base for learning about these concepts.
func calculateArea(height: Int, width: Int) -> Int {
return height * width
}
let area = calculateArea(100, 200) //-> 200
So as we've learned this is our typical run-of-the-mill function that contains two parameters, height
and width
; it also contains a return value which is required to be a signed integer value. Inside our function we have a simple return
statement which multiplies the height
by the width
.
Pretty simple, yep.
2. What it looks like with parameter labels, now how does it behave?
So unlike our standard function above which doesn't require anything other than two integer values as arguments we need to declare labels with them if we do use them.
func calculateArea(myHeight h: Int, myWidth w: Int) -> Int {
return h * w
}
let area = calculateArea(myHeight: 100, myWidth: 200) //-> 200
So... If you're confused because of the example Amit showed in the video don't fear as the label we define can be anything. When the Swift compiler sees our parameter labels it changes the function signature requiring them to be declared when passing in our arguments.
One other thing you will notice is instead of height
and width
as the parameter names they are now h
and w
, this is only an example but it shows how labels can take away some control from how we name our parameters allowing for much easier to read function signatures.
3. But wait, labels are only half the story
Another technique shown in the video are named parameters, unlike parameters these define an explicit function signature that can't be changed in anyway meaning you need to have parameters that are clearly named to prevent unexpected results when calling your function.
func calculateArea(#height: Int, #width: Int) -> Int {
return height * width
}
let area = calculateArea(height: 100, width: 200) //-> 200
So what changed? Aside from adding a hash/pound symbol before height
and width
absolutely nothing. This example still behave the same way to how our parameter labels do with the exception that you can only change the parameter names to give more meaning to the arguments which 99% of the time can be accomplished using standard naming conventions.
What's next?
Try each technique, see which one fits best in your workflow.
Hope that helps.

gustavopares
Courses Plus Student 30 PointsSo there are 3 techniques a basic function with parameters, a function with labels, and a function with named parameters
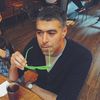
Micky Hingorani
5,437 PointsThank you, Chris, for your response. I had the same question and found your thoughts helpful. However, I still wasn't 100% clear on everything.
Looking into it further, the main point that I needed to hear a couple of times is that you need external parameter labels when order is important. Amit touches on that but seeing the same example twice where order doesn't matter, simple multiplication when calculating area, had me confused. I just didn't really see the difference, and hence the value.
For anyone looking for more, I found this explanation online clear and helpful.
Richard Levy
554 PointsRichard Levy
554 PointsThanks for your detailed response Chris. I also read through Apple's material on functions and I think I've got it straight. A "named parameter" is simply a parameter with an external name (perhaps you can correct me if I'm wrong).
I think I was thrown off when Amit used the term label. I thought label was a term with a specific technical meaning, different than parameter name. But if I understand it correctly, Amit - and you - use the term label somewhat interchangeably with the term parameter name.
Thanks again.
Chris Shaw
26,676 PointsChris Shaw
26,676 PointsNo, named parameters as I mentioned above in my third point use the hash/pound symbol which refers the label to the local parameter naming meaning it can't be changed without renaming the parameter name itself, external parameters names or parameter label as it's also known as and described in detail in my second point is the use of a word or collection of words in camel case to provide a more details description of what the parameter is for hence my second example where the parameters become
h
andw
because they have labels to describe them.Don't worry if it still seems confusing, there is no requirement around using these constantly but they are recommended in places where there's little context or a lack of documentation.